Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial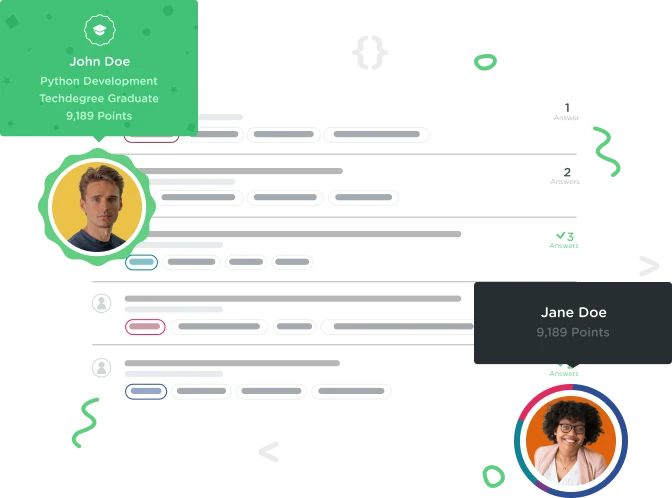

Braeden Long
2,137 PointsI would love some feedback on my Reversed Numbers Game!
Hi everyone! I've been taking the Python Basics course and was taught how to make a simple Numbers Game in which I try to guess a random number the computer generated within a range of two numbers. I was challenged to make a Reversed Numbers Game in which I think of a number and the computer attempts to guess it. I made it interesting by offering the computer hints of going lower or higher. The most challenging part for me was making sure the computer did not guess the same number twice. It took me a while, but I determined that using a list would be very helpful. Please review the code and let me know if there is any way I can shorten or simplify the program. I only ask two things: be constructive in your criticism, and keep in mind my knowledge of Python's capabilities are limited.
attempts = 15
low_num = 1
high_num = 100
def game_rules():
print("""Let's play the Numbers Game!
You pick a number between {} and {}, and I'll have {} chances to guess what you're thinking of!
If I get it right, type 'Y'
If I need to go lower, type 'L'
If I need to go higher, type 'H'
Good luck!
""".format(low_num, high_num, attempts))
def reversed_numbers_game():
count = 0
import random
guess_list = [low_num, high_num]
computer_guess = random.randint(guess_list[0], guess_list[1])
def victory():
print("Woohoo! I did it!")
def defeat():
print("Is your number... Oh no! I've run out of guesses!")
while count < attempts:
guess = input("Is {} your number? (Y/H/L): ".format(computer_guess))
if guess.upper() == "Y":
victory()
break
elif guess.upper() == "H":
guess_list[0] = computer_guess + 1
if guess_list[0] - guess_list[1] == 0:
print("Your number must be {}!".format(computer_guess))
victory()
break
elif guess_list[0] - guess_list[1] == 1:
print("I can't go higher! Let's try again.")
break
computer_guess = random.randint(guess_list[0], guess_list[1])
elif guess.upper() == "L":
guess_list[1] = computer_guess - 1
if guess_list[0] - guess_list[1] == 0:
print("Your number must be {}!".format(computer_guess))
victory()
break
elif guess_list[0] - guess_list[1] == 1:
print("I can't go lower! Let's try again.")
break
computer_guess = random.randint(guess_list[0], guess_list[1])
else:
print("I don't understand your input. Please try again.")
continue
count += 1
else:
defeat()
game_rules()
while True:
begin_game = input("Think of a number... Are you ready? (Y/N): ")
if begin_game.upper() == "Y":
reversed_numbers_game()
elif begin_game.upper() == "N":
break
else:
print("I don't understand your input.")
continue