Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial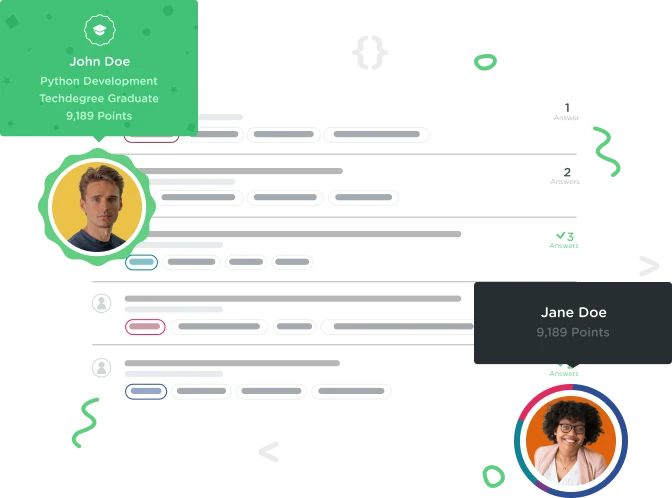

Mithun B
1,978 PointsI wrote a program to show the days, minutes and seconds a person lived till his age
var secondsInMinute = 60;
var minutesInHour = 60;
var hoursInDay = 24;
var daysInYear = 365;
var myName = prompt("What is your name?");
var myAge = prompt("What is your age?");
var secondsInHour = secondsInMinute * minutesInHour;
var secondsInDay = secondsInHour * hoursInDay;
var totalDaysAlive = myAge * daysInYear;
var totalHoursAlive = hoursInDay * totalDaysAlive;
var totalMinutesAlive = totalHoursAlive * minutesInHour;
var totalSecondsAlive = totalMinutesAlive * secondsInMinute;
document.write(myName + ", You lived for:<b> " + totalDaysAlive + " </b>days<br/>");
document.write(myName + ", You lived for:<b> " + totalHoursAlive + " </b>Hours <br/>");
document.write(myName + ", You lived for:<b> " + totalMinutesAlive + " </b>Hours <br/>");
document.write(myName + ", You lived for:<b> " + totalSecondsAlive + " </b>Seconds <br/>");
Is this write?
I also wrote some CSS for this program and it looks cool. Tell me if you find any mistakes in this program.
PS: I am loving JavaScript. It's different from PHP and users don't even need to refresh the page :D
Thanks
3 Answers

Chris Shaw
26,676 PointsHi Mithun,
The only issue I see is the following line as when a user enters their age it will be a string so to ensure 100% consistency it's better to parse it first as an integer.
var totalDaysAlive = parseInt(myAge, 10) * daysInYear;
One thing I would just from a fail safe point of view is add error checking to both your prompts to ensure a valid name and valid age were entered which will ensure your code doesn't break and cause issues.
var myName = prompt("What is your name?");
if (!myName) {
alert("You didn't enter a valid name");
return false;
}
var myAge = prompt("What is your age?"),
myAge = parseInt(myAge, 10);
if (isNaN(myAge)) {
alert("You didn't enter a valid age");
return false;
}
You can get a lot more advanced with these kinds of checks but I wanted to keep it simple.

Mithun B
1,978 PointsThanks a lot, Chris. I will definitely keep this in mind.
Thanks again, Mithun
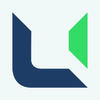
Leon Laci
Courses Plus Student 4,423 PointsWhat about 366 days in a year?

Chris Shaw
26,676 PointsI found a nice little hack on Stack Overflow which you can use to determine if it's a leap year.
var isLeapYear = new Date(new Date().getFullYear(), 1, 29).getMonth() === 1;
var daysInYear = isLeapYear ? 366 : 365;
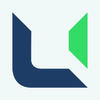
Leon Laci
Courses Plus Student 4,423 PointsThanks Chris
moath alqutoob
2,450 Pointsmoath alqutoob
2,450 PointsChris Upjohn can you pleas explane me this cod
var totalDaysAlive = parseInt(myAge, 10) * daysInYear; what the different between this ; var totalDaysAlive = myAge * daysInYear;
2-and i wont explan this
var myName = prompt("What is your name?");
if (!myName) { alert("You didn't enter a valid name"); return false; }
var myAge = prompt("What is your age?"), myAge = parseInt(myAge, 10);
if (isNaN(myAge)) { alert("You didn't enter a valid age"); return false; }
Chris Shaw
26,676 PointsChris Shaw
26,676 PointsHi moath alqutoob,
The reason for using
parseInt
is because theprompt
function will always return a string therefore we may not get a consistent result between browsers if we don't first parse the given value as a number which eliminates any inconsistencies.