Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial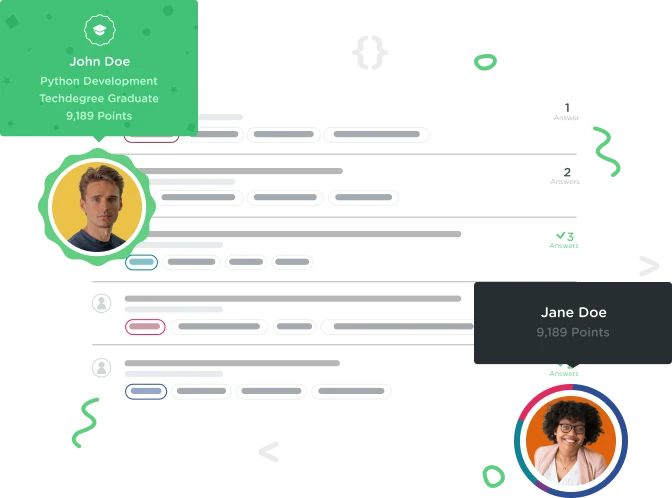

Blaize Lange
2,298 PointsI wrote my code challenge a little different and don't understand why it won't work.
I see how Dave put each variable with the if condition. I just did all the variables and then all the conditions, however when I run mine at the end only if I get everyone correct it reads the first one but seems to skip the two else if causes I wrote and only registers all correct or none correct. So it doesn't register if I got 2-4 questions correct or 1 correct. If someone can explain what is wrong with what I wrote I would appreciate it. The more detail the better, thank you! p.s. If putting the variable with the condition is the only way to do it then way does it read if I answer all 5 correct? Also, it is space corrected just when I copy/paste the code to this questions box it doesn't separate the lines of code, I don't know why sorry about that.
// correctAnswer var + questions asked
var correctAnswer = 0;
var question1 = prompt('Is the moon blue?');
var question2 = prompt('Is grass green?');
var question3 = prompt('Do planes fly?');
var question4 = prompt('Can cars drive fast?');
var question5 = prompt('Is the ocean orange?');
// add + 1 to correctAnswer var if answer = yes or no
if (question1.toLowerCase === 'no'){
correctAnswer += 1;
}
if (question2.toLowerCase === 'yes'){
correctAnswer += 1;
}
if (question3.toLowerCase === 'yes'){
correctAnswer += 1;
} if (question4.toLowerCase === 'yes'){
correctAnswer += 1;
}
if (question5.toLowerCase === 'no'){
correctAnswer += 1;
}
// How many correct message
if (correctAnswer === 5) {
document.write('You got 5 correct answers and receive a gold star!');
} else if ( correctAnswer >= 2) {
document.write('You got 2 - 4 questions correct and receive a silver star!');
} else if( correctAnswer >= 1) {
document.write('You only got one correct answer and receive a bronze star!');
} else {
document.write('You got no correct answers and do not receive any stars :(');
}
3 Answers

andren
28,558 PointsThe problem with your code is that when you call a function you have to have a pair of parenthesis at the end of it, like this "toLowerCase()" if you don't then JavaScript won't actually run the function but will instead treat it as a reference to the function itself.
So in your if statements you are not comparing a lower cased version of the question answer to the question solution, you are instead comparing the "toLowerCase" function itself against the answer solution, which means that none of the code in the if statements will ever run, regardless of the answers you enter.
If you add the parenthesis like this:
// correctAnswer var + questions asked
var correctAnswer = 0;
var question1 = prompt('Is the moon blue?');
var question2 = prompt('Is grass green?');
var question3 = prompt('Do planes fly?');
var question4 = prompt('Can cars drive fast?');
var question5 = prompt('Is the ocean orange?');
// add + 1 to correctAnswer var if answer = yes or no
if (question1.toLowerCase() === 'no'){
correctAnswer += 1;
}
if (question2.toLowerCase() === 'yes'){
correctAnswer += 1;
}
if (question3.toLowerCase() === 'yes'){
correctAnswer += 1;
}
if (question4.toLowerCase() === 'yes'){
correctAnswer += 1;
}
if (question5.toLowerCase() === 'no'){
correctAnswer += 1;
}
// How many correct message
if (correctAnswer === 5) {
document.write('You got 5 correct answers and receive a gold star!');
} else if ( correctAnswer >= 2) {
document.write('You got 2 - 4 questions correct and receive a silver star!');
} else if( correctAnswer >= 1) {
document.write('You only got one correct answer and receive a bronze star!');
} else {
document.write('You got no correct answers and do not receive any stars :(');
}
Then your code will work fine.
It is worth noting that with the code you posted it is impossible to ever get any result other than the "no correct answers" response. So I have no idea how you ended up with a different response when you entered all of the answers correctly, did you make some small change to the code between that test and the version you posted here perhaps? Either way with the fix I posted above your code will work perfectly fine.
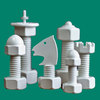
Steven Parker
231,269 Points
When you call a method, you must include parentheses even if it takes no argument.
So for your case-insensitive comparisons to work, you need to add parentheses to the toLowerCase calls:
if (question1.toLowerCase() === 'no')
To get your code to appear properly you must format it using the instructions in the Markdown Cheatsheet pop-up found below the answer area on this page

Blaize Lange
2,298 PointsAwesome thank you guys for your help. I feel like I am going to be making a lot of little mistakes like this starting off, its nice to have the help! And thank you for the Markdown tip, this is the first time I posted code to a question so I didn't know and will definitely use it in the future!