Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial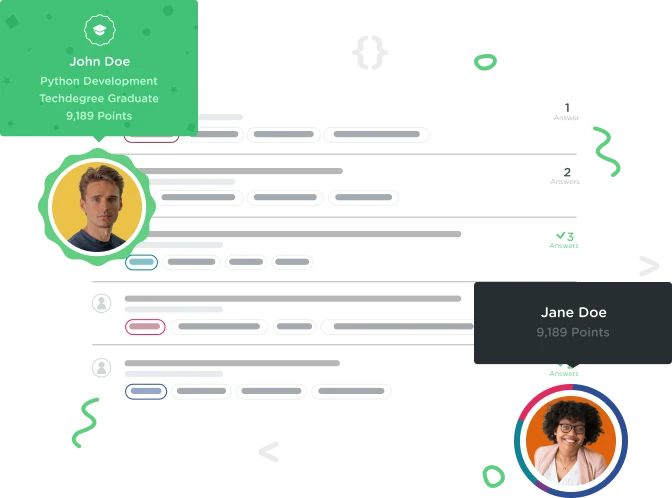

Dávid Molnár
27,081 PointsI wrote the comparer method but it seems like it does nothing. (added * -1)
class Program {
static void Main(string[] args)
{
string currDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directory = new DirectoryInfo(currDirectory);
var fileName = Path.Combine(directory.FullName, "players.json");
var players = DeserializePlayers(fileName);
var topTenPlayers = GetTopTenPlayers(players);
foreach (var player in topTenPlayers)
{
Console.WriteLine("{0} : {1}", player.FirstName, player.PointPerGame);
}
fileName = Path.Combine(directory.FullName, "topten.json");
SerializePlayersToFile(topTenPlayers, fileName);
Console.ReadKey();
}
public static List<Player> DeserializePlayers(string fileName)
{
var players = new List<Player>();
var serializer = new JsonSerializer();
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
players = serializer.Deserialize<List<Player>>(jsonReader);
}
return players;
}
public static List<Player> GetTopTenPlayers(List<Player> players)
{
var topTenPlayers = new List<Player>();
players.Sort(new PlayerComparer());
int counter = 0;
foreach (var player in players)
{
topTenPlayers.Add(player);
counter++;
if (counter == 10)
break;
}
return topTenPlayers;
}
public static void SerializePlayersToFile(List<Player> players, string fileName)
{
var serializer = new JsonSerializer();
using (var writer = new StreamWriter(fileName))
using (var jsonWriter = new JsonTextWriter(writer))
{
serializer.Serialize(jsonWriter, players);
}
}
}
}
public class PlayerComparer : IComparer<Player>
{
public int Compare(Player x, Player y)
{
return x.PointPerGame.CompareTo(y.PointPerGame) * -1;
}
}
Everything else works correctly but this one. This method should sort the list from the maximum values to the lowest ones, add the top ten values to the new list and finally write their "FirstName" and "PointPerGame" to the console but when I run it, all I see is 0 points.
(I created a new individual class for the PlayerComparer class.)
Brendan Bates
2,264 PointsBrendan Bates
2,264 Pointsinstead of using the operation * -1 I used swapped the x and y variables in the CompareTo operation and it seemed to work a lot better.
return y.PointsPerGame.CompareTo(x.PointsPerGame);
instead of
return x.PointsPerGame.CompareTo(y.PointsPerGame) * -1;