Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial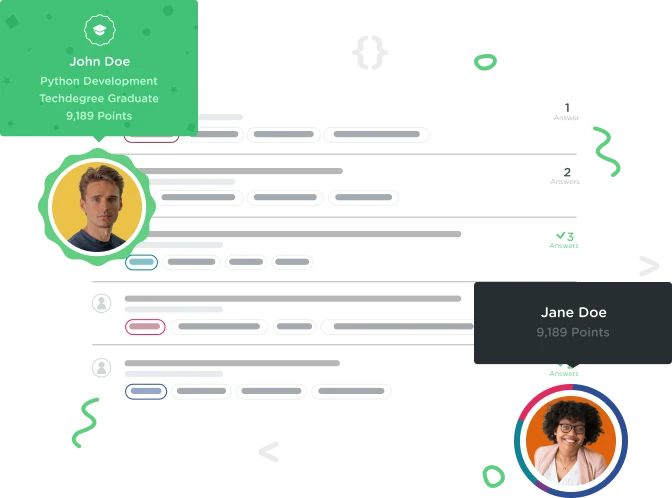

Azim Sodikov
11,432 PointsIam getting this error, cant debug
ERROR in missing ) after argument list @ ./app.js 17:0-26 webpack: Failed to compile.
even though I just copy and pasted the project files!
4 Answers

matth89
17,826 PointsHi, can you post your actual code? I may be able to better assist you that way

Azim Sodikov
11,432 Pointsthe error is coming from bundle.js and its 22000 line

Azim Sodikov
11,432 Pointsso I copied and pasted final project files and run npm install and install all stuff, and run npm start and terminal gives me this above errors while compiling the file!

Azim Sodikov
11,432 Points// Libs
import React from 'react';
const INITIAL_STATE = {
players: [
{
name: "Jim Hoskins",
score: 31,
},
{
name: "Andrew Chalkley",
score: 20,
},
{
name: "Alena Holligan",
score: 50,
},
],
}
const Scoreboard = React.createClass({
getInitialState: function () {
return INITIAL_STATE;
},
onScoreChange: function (index, delta) {
this.state.players[index].score += delta;
this.setState(this.state);
},
onAddPlayer: function (name) {
this.state.players.push({ name: name, score: 0 });
this.setState(this.state);
},
onRemovePlayer: function (index) {
this.state.players.splice(index, 1);
this.setState(this.state);
},
render: function () {
return (
<div className="scoreboard">
<Header players={this.state.players} />
<div className="players">
{this.state.players.map(function(player, index) {
return (
<Player
name={player.name}
score={player.score}
key={player.name}
onScoreChange={(delta) => this.onScoreChange(index, delta)}
onRemove={() => this.onRemovePlayer(index)}
/>
);
}.bind(this))}
</div>
<AddPlayerForm onAdd={this.onAddPlayer} />
</div>
);
}
});
function Header(props) {
return (
<div className="header">
<Stats players={props.players} />
<h1>Scoreboard</h1>
<Stopwatch />
</div>
);
}
Header.propTypes = {
players: React.PropTypes.array.isRequired,
};
function Stats(props) {
const playerCount = props.players.length;
const totalPoints = props.players.reduce(function(total, player) {
return total + player.score;
}, 0);
return (
<table className="stats">
<tbody>
<tr>
<td>Players:</td>
<td>{playerCount}</td>
</tr>
<tr>
<td>Total Points:</td>
<td>{totalPoints}</td>
</tr>
</tbody>
</table>
)
}
Stats.propTypes = {
players: React.PropTypes.array.isRequired,
};
const Stopwatch = React.createClass({
getInitialState: function () {
return ({
running: false,
previouseTime: 0,
elapsedTime: 0,
});
},
componentDidMount: function () {
this.interval = setInterval(this.onTick);
},
componentWillUnmount: function () {
clearInterval(this.interval);
},
onStart: function () {
this.setState({
running: true,
previousTime: Date.now(),
});
},
onStop: function () {
this.setState({
running: false,
});
},
onReset: function () {
this.setState({
elapsedTime: 0,
previousTime: Date.now(),
});
},
onTick: function () {
if (this.state.running) {
var now = Date.now();
this.setState({
elapsedTime: this.state.elapsedTime + (now - this.state.previousTime),
previousTime: Date.now(),
});
}
},
render: function () {
var seconds = Math.floor(this.state.elapsedTime / 1000);
return (
<div className="stopwatch" >
<h2>Stopwatch</h2>
<div className="stopwatch-time"> {seconds} </div>
{ this.state.running ?
<button onClick={this.onStop}>Stop</button>
:
<button onClick={this.onStart}>Start</button>
}
<button onClick={this.onReset}>Reset</button>
</div>
)
}
});
function Player(props) {
return (
<div className="player">
<div className="player-name">
<a className="remove-player" onClick={props.onRemove}>ā</a>
{props.name}
</div>
<div className="player-score">
<Counter onChange={props.onScoreChange} score={props.score} />
</div>
</div>
);
}
Player.propTypes = {
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
onRemove: React.PropTypes.func.isRequired,
onScoreChange: React.PropTypes.func.isRequired,
};
function Counter(props) {
return (
<div className="counter" >
<button className="counter-action decrement" onClick={() => props.onChange(-1)}>
-
</button>
<div className="counter-score"> {props.score} </div>
<button className="counter-action increment" onClick={() => props.onChange(1)}>
+
</button>
</div>
);
}
Counter.propTypes = {
onChange: React.PropTypes.func.isRequired,
score: React.PropTypes.number.isRequired,
}
const AddPlayerForm = React.createClass({
propTypes: {
onAdd: React.PropTypes.func.isRequired,
},
getInitialState: function () {
return { name: "" };
},
onNameChange: function (e) {
const name = e.target.value;
this.setState({ name: name });
},
onSubmit: function (e) {
if (e) e.preventDefault();
this.props.onAdd(this.state.name);
this.setState({ name: "" });
},
render: function () {
return (
<div className="add-player-form">
<form onSubmit={this.onSubmit}>
<input
type="text"
value={this.state.name}
onChange={this.onNameChange}
placeholder="Player Name"
/>
<input type="submit" value="Add Player" />
</form>
</div>
);
}
});
export default Scoreboard;

Azim Sodikov
11,432 Pointsthis is the source code
Azim Sodikov
11,432 PointsAzim Sodikov
11,432 PointsI posted the actual code btw!