Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial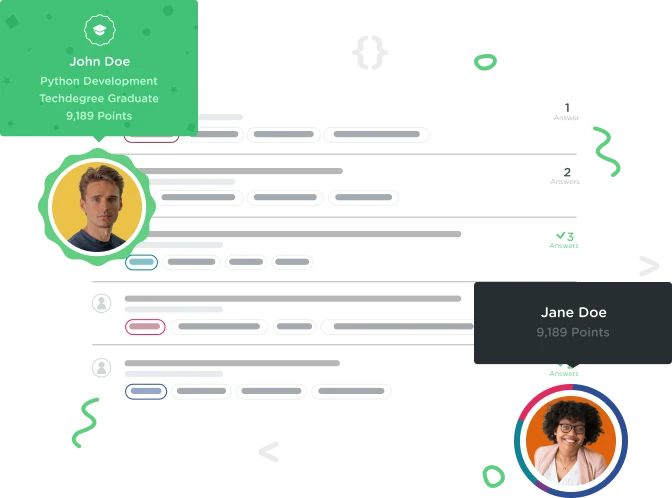

Joe Worthington
1,329 PointsI'd like some critique and suggestions for improvement on my Quiz game please.
/*The Quiz Game
Answer five questions
Win a prize
By Joe Worthington
*/
//Welcomes player, asks for name and then displays the the ranking
var player = prompt("Hello and welcome to The Quiz Game. Here are 5 questions to answer. Let's begin with your name?");
document.write("<p>" + player + " here is what you can win:</p>" + "<p>5 correct answers = GOLD badge</p>" + "<p>3-4 correct answers = SILVER badge</p>" + "<p>1-2 correct answers = BRONZE badge</p>" + "<p>0 correct answers = THE DUNCE'S HAT</p>");
//Question 1
var playerAnswer1 = prompt("Question 1. Who wrote The New York Trilogy?");
var answer1 = "paul auster".toUpperCase() ;
if (playerAnswer1.toUpperCase() === answer1) {
var score = 1;
} else (score = 0); {
document.write("<p>Your answer was " + playerAnswer1 + "</p>");
}
//Prints score to console log
console.log(score);
//Question 2
var playerAnswer2 = prompt("Question 2. What artist painted the picture Guernica?");
var answer2 = "picasso".toUpperCase();
if (playerAnswer2.toUpperCase() === answer2) {
score = score + 1;
} else ( score + 0); {
document.write("<p>Your answer was " + playerAnswer2) + "</p>";
}
//Prints score to console log
console.log(score);
//Question 3
var playerAnswer3 = prompt("Question 3. What is 10% of 35?");
var answer3 = 3.5;
if (parseFloat(playerAnswer3) === answer3) {
score = score + 1;
} else (score + 0); {
document.write("<p>Your answer was " + playerAnswer3 + "</p>");
}
//Prints score to console log
console.log(score);
//Question 4
//Assumes the answer is false
var correctAnswer4 = false
//Generates the random number I'm thinking of between 1-10
var randomNumber = Math.floor(Math.random() * 10) +1;
/*User has first attempt with the following results:
1.Guess it right first time and it writes the guess to the document
2.Second attempt with a hint as to the random number being higher or lower
3. Prints the second attempt to the document
*/
var playerAnswer4 = prompt("Question 4. What number am I thinking of between 1-10? (it's okay, you get two attempts!)");
if (parseInt(playerAnswer4) === randomNumber ) {
correctGuess = true;
score = score + 1;
document.write("<p>Your answer was " + playerAnswer4 + ", right first time, you lucky son-of-a-gun!");
} else if (parseInt(playerAnswer4) < randomNumber) {
var guessMore = prompt('Try again. The number I was thinking of is more than ' + playerAnswer4);
if (parseInt(guessMore) === randomNumber) {
correctAnswer4 = true;
score = score + 1;
document.write("<p>Your first guess was " + guessMore);
} else (score + 0); {
document.write("<p>Your second guess was " + guessMore);
}
} else if ( parseInt(playerAnswer4) > randomNumber) {
var guessLess = prompt('Try again. The number I was thinking of is less than '+ playerAnswer4);
if (parseInt(guessLess) === randomNumber) {
correctAnswer4 = true;
score = score + 1;
document.write("<p>Your first guess was " + guessLess);
} else (score + 0); {
document.write("<p>Your second guess was " + guessLess);
}
}
//Prints score to console log
console.log(score);
//Question 5
var playerAnswer5 = prompt("Danny's mother has four children...Jimmy, Jason and Rachel... and what's the name of the fourth child?");
var answer5 = "danny".toUpperCase();
if (playerAnswer5.toUpperCase() === answer5) {
score = score + 1;
} else (score + 0); {
document.write("<p>Your answer was " + playerAnswer5);
}
//Prints score to console log
console.log(score);
alert("So, how did you do? Let's find out.");
if (score === 5) {
document.write("<h1>You scored " + score + "! You won a GOLD badge! Congratulations " + player + ".</h1>");
} else if (score === 4 || score === 3) {
document.write("<h2>You scored " + score + "! You won a SILVER badge! Well done " + player + ".</h2>");
} else if (score === 2 || score === 1) {
document.write("<h3>You scored " + score + "! You won a BRONZE badge! Not bad " + player + ".</h3>");
} else (document.write("<h4>Your score was " + score + ". Hm, " + player + " you're going to have to wear THE DUNCE'S HAT. Maybe give it another go, hey!</h4>")); {
}
//Prints score to console log
console.log(score);
3 Answers
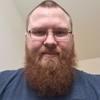
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsIf I remember correct - you don't need to write else (score = 0); because the "else" is a back-up path for your program if your "if and else if" is false - you can simply just write else { } and it will do the same thing..
but again, I might be wrong..
I really like how you made the QUIZ though - really good job :)
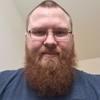
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsIf I play the game I would get 2-3 correct answers depending on if I can guess the "guess number" question :)
I really like the variety of your questions because when I made the game I just made 5 questions about capitals in different countries :)

Joe Worthington
1,329 PointsThanks very much Henrik.

Nathan Wakefield
7,065 PointsI recommend printing a little information about the score as you log it. Several places in your code you have
console.log(score);
This is great, especially for debugging. However, it would be even better if you printed something to identify what the score corresponds to: for instance,
console.log('Score after Question 3: ' + score);
or even something shorter if that's too long for your tastes. Just so long as you can quickly recognize what the score corresponds to.

Joe Worthington
1,329 PointsHi Nathan,
Thanks very much for the feedback. I think you're right.
I can see the benefit for sure.
Cheers
Joe
Joe Worthington
1,329 PointsJoe Worthington
1,329 PointsHi Henrik,
Thanks for the feedback. I just started watching the solution to the challenge and the first thing Dave McFarland says is to create a variable with a score of 0. This explains your suggestion of not needing to write the else (score = 0).
I think establishing the empty score variable to start is the better way for sure, so thanks for your suggestion.
What did you win? (Just out of curiosity!)