Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial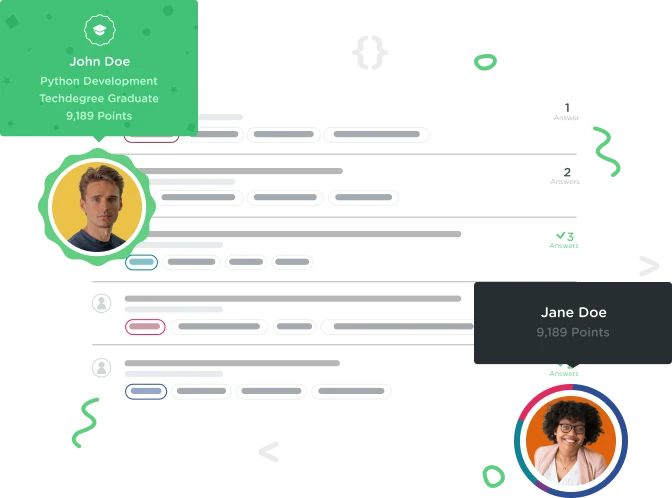
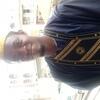
Temitope Ogunleye
Full Stack JavaScript Techdegree Student 2,186 PointsI'd like some feedback on my code. Is there any parts I could improve on? I'm happy with my outcome regardless!
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
let correct = 0;
// 2. Store the rank of a player
let rank;
// 3. Select the <main> HTML element
const main = document.querySelector('main');
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
// Storing Each Answer in a Variable
const correctOne = 'PYTHON';
const correctTwo = 'RUBY';
const correctThree = 'CSS';
const correctFour = 'HTML';
const correctFive = 'JAVASCRIPT';
// FIVE QUESTIONS
const pythonAnswer = prompt("Name a programming language that's also a snake");
if (pythonAnswer.toUpperCase() === correctOne ) {
correct += 1;
}
const rubyAnswer = prompt("Name a programming language that's also a gem");
if (rubyAnswer.toUpperCase() === correctTwo) {
correct += 1;
}
const cssAnswer = prompt("What programming language do you use to style web pages");
if (cssAnswer.toUpperCase() === correctThree ) {
correct += 1;
}
const htmlAnswer = prompt("What language do you use to build structure of web ages");
if (htmlAnswer.toUpperCase() === correctFour ) {
correct += 1;
}
const javascriptAnswer = prompt("What language do you use to add interactivity to a web age?");
if (javascriptAnswer.toUpperCase() === correctFive ) {
correct += 1;
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if (correct === 0) {
rank = 'No Crown'
} else if (correct === 1) {
rank = 'Bronze'
} else if (correct === 2) {
rank = 'Bronze'
} else if (correct === 3 ) {
rank = 'Silver'
} else if (correct === 4 ) {
rank = 'silver'
} else {
rank = 'Gold'
}
// 6. Output results to the <main> element
main.innerHTML = `<h2>You got ${correct} out of 5 questions correct</h2>
<p>Crown earned: <strong>${rank}</strong></p>`;
1 Answer
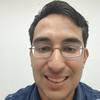
Jesús Armenta
13,505 PointsVery nice work! I like it's well documented and it's easy to follow what is the code doing, there are some areas of opportunity like I would rename some variables like 'correct' to 'correctAnswers' and instead of 'correctOne' maybe 'firstAnswer'. The if else chain can be replace with a switch.
Also the next suggestion depend on whether you have knowledge of arrays and for cycles, you can put each message to prompt and the correct answer in an object array and iterate it to reduce the number of variables needed and also make use of functions to wrap code that will need be called several times.
In my experience I can tell that this is good to go, you should focus on learning the fundamentals and once you start learning something new make sure to practice a lot to master it, maybe today is not making sense but learning tomorrow can be different.
Temitope Ogunleye
Full Stack JavaScript Techdegree Student 2,186 PointsTemitope Ogunleye
Full Stack JavaScript Techdegree Student 2,186 PointsThank you very much. I was able to use switches, and changed better names. I'll continue the rest of the full stack path to learn functions and arrays.