Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial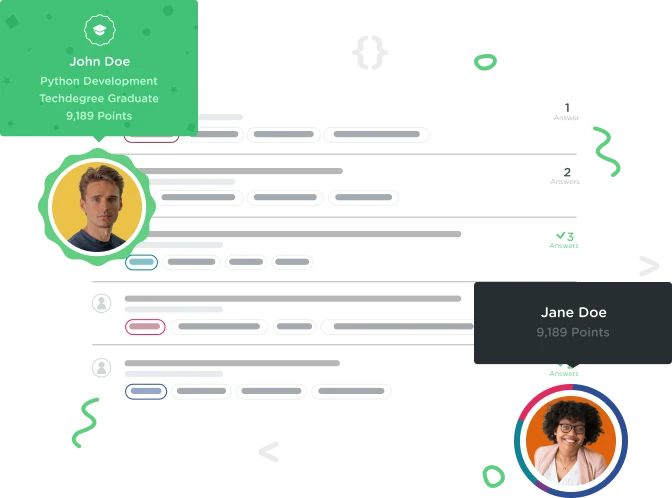

Gabriel Rafi
690 PointsI'd like some feedback on my code. Is there any parts I could improve on? I'm happy with my outcome regardless! :D
I'd lik some feedback on the conditional challenge I came up with without using guils video to guide me. It isnt identical but I'm happy with the outcome considering this is my first time ever studying code. I could've used the >= sign but before watching the solution I thought it might take the 5 and include it in the >= 3.
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
let questionOne = prompt("true/false - A snowcone is an icecream. (Don't use capital letters)");
let questionTwo = prompt("True/False - A snowcone is an icecream. (Don't use capital letters)");
let questionThree = prompt("True/False - A snowcone is an icecream. (Don't use capital letters)");
let questionFour = prompt("True/False - A snowcone is an icecream. (Don't use capital letters)");
let questionFive = prompt("True/False - A snowcone is an icecream. (Don't use capital letters)");
// 2. Store the rank of a player
let playerRank = 0;
let crownRank = '';
// 3. Select the <main> HTML element
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
if ( questionOne == "false" ) {
playerRank += +1;
// console.log(`Your score is ${playerRank}`);
} else {
playerRank += 0;
// console.log(`Your score is ${playerRank}`);
}
if ( questionTwo == "false" ) {
playerRank += +1;
// console.log(`Your score is ${playerRank}`);
} else {
playerRank += 0;
// console.log(`Your score is ${playerRank}`);
}
if ( questionThree == "false" ) {
playerRank += +1;
// console.log(`Your score is ${playerRank}`);
} else {
playerRank += 0;
// console.log(`Your score is ${playerRank}`);
}
if ( questionFour == "false" ) {
playerRank += +1;
// console.log(`Your score is ${playerRank}`);
} else {
playerRank += 0;
// console.log(`Your score is ${playerRank}`);
}
if ( questionFive == "false" ) {
playerRank += +1;
console.log(`Your score is ${playerRank}`);
} else {
playerRank += 0;
console.log(`Your score is ${playerRank}`);
}
if ( playerRank === 5 ) {
console.log(`You've earned the GOLD crown.`);
crownRank = "Gold";
} else if ( playerRank === 4 || playerRank === 3 ) {
console.log(`You've earned the Silver crown.`);
crownRank = "Silver";
} else if ( playerRank === 2 || playerRank === 1 ) {
console.log(`You've earned the Bronze crown.`);
crownRank = "Bronze";
} else {
console.log(`You've earned no crown because you did so poorly...`);
crownRank = "No crown";
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
// 6. Output results to the <main> element
document.write();
document.querySelector('main').innerHTML = "Your rank was" + " " + playerRank + " " + "(" + crownRank + ")" + " " + "<-- That is the crown you've received";
2 Answers
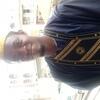
Temitope Ogunleye
Full Stack JavaScript Techdegree Student 2,186 Points/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
let correct = 0;
// 2. Store the rank of a player
let rank;
// 3. Select the <main> HTML element
const main = document.querySelector('main');
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
// Storing Each Answer in a Variable
const correctOne = 'PYTHON';
const correctTwo = 'RUBY';
const correctThree = 'CSS';
const correctFour = 'HTML';
const correctFive = 'JAVASCRIPT';
// FIVE QUESTIONS
const pythonAnswer = prompt("Name a programming language that's also a snake");
if (pythonAnswer.toUpperCase() === correctOne ) {
correct += 1;
}
const rubyAnswer = prompt("Name a programming language that's also a gem");
if (rubyAnswer.toUpperCase() === correctTwo) {
correct += 1;
}
const cssAnswer = prompt("What programming language do you use to style web pages");
if (cssAnswer.toUpperCase() === correctThree ) {
correct += 1;
}
const htmlAnswer = prompt("What language do you use to build structure of web ages");
if (htmlAnswer.toUpperCase() === correctFour ) {
correct += 1;
}
const javascriptAnswer = prompt("What language do you use to add interactivity to a web age?");
if (javascriptAnswer.toUpperCase() === correctFive ) {
correct += 1;
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if (correct === 0) {
rank = 'No Crown'
} else if (correct === 1) {
rank = 'Bronze'
} else if (correct === 2) {
rank = 'Bronze'
} else if (correct === 3 ) {
rank = 'Silver'
} else if (correct === 4 ) {
rank = 'silver'
} else {
rank = 'Gold'
}
// 6. Output results to the <main> element
main.innerHTML = `<h2>You got ${correct} out of 5 questions correct</h2>
<p>Crown earned: <strong>${rank}</strong></p>`;

Jason Cheung
3,227 Pointslet score = 0;
let crown = 0;
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
//Questions to ask
const answer1 = prompt("What programming language used for styling a webpage")
const answer2 = prompt("What language are frequently used in websites besides HTML and CSS")
const answer3 = prompt("What language is minecraft using")
const answer4 = prompt("Which programming language have a snake as the logo")
const answer5 = prompt("Which programming language mainly built Mirosoft Windows")
//default answers
const correctAnswer1 = "CSS";
const correctAnswer2 = "JAVASCRIPT";
const correctAnswer3 = "JAVA";
const correctAnswer4 = "PYTHON";
const correctAnswer5 = "C#";
//Crowns Earned
const goldCrown = "Gold Crown";
const silverCrown = "Silver Crown";
const bronzeCrown = "Bronze Crown";
const noCrown = "No Sh*t Was Given To You";
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if (answer1.toUpperCase() === correctAnswer1){
score+=1
};
if (answer2.toUpperCase() === correctAnswer2){
score+=1
};
if (answer3.toUpperCase() === correctAnswer3){
score+=1
};
if (answer4.toUpperCase() === correctAnswer4){
score+=1
};
if (answer5.toUpperCase() === correctAnswer5){
score+=1
};
if (score === 5){
crown = goldCrown
}else if (+score >= 3){
crown = silverCrown
}else if (+score >= 1){
crown = bronzeCrown
}else{
crown = noCrown
}
// 6. Output results to the <main> element
const result = `<h2>You got ${score} out of 5</h2>
<p>Crown Earned: ${crown}</p>`
document.querySelector("main").innerHTML = result;
Jason Cheung
3,227 PointsJason Cheung
3,227 Pointsyou could have chose not to include some of the else statements as it is adding 0 to the variable. you could also have used .toUpperCase so that the user could type either lower and upper case.