Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial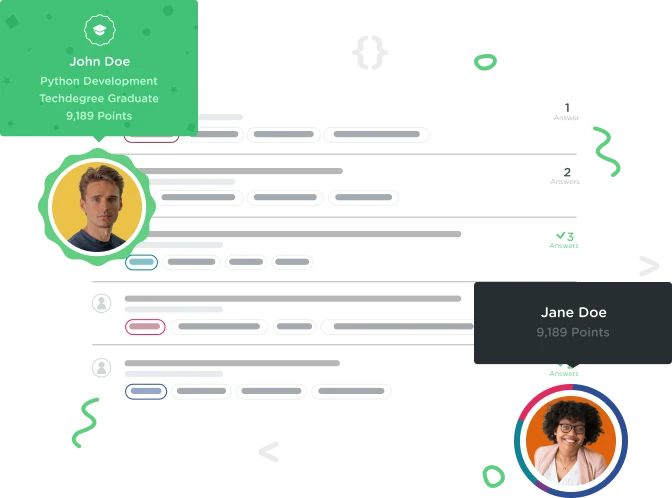

assalbiswa
6,048 Pointsidentical()
<script>
var myUndefinedVariable;
var myNullVariable = null;
if(myNullVariable == myUndefinedVariable) {
identical();
}
</script>
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Variables</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
<script src="ignore_this.js"></script>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Variables: Null and Undefined</h2>
<div id="container">
</div>
<script>
var myUndefinedVariable;
var myNullVariable = null;
if(myNullVariable == myUndefinedVariable) {
identical();
}
</script>
</body>
</html>
1 Answer
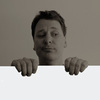
Sean T. Unwin
28,690 PointsThere is a great explanation by Erik McClintock in this thread. For brevity I will paste his answer here. All credit is to Erik so feel free to upvote his post in the linked thread.
"
The challenge is telling you that the identical method is being called, despite the fact that the values being checked in the conditional are not identical (and the purpose of that conditional is to verify that the two values being compared are, in fact, identical before allowing the identical method to run). The challenge wants you to correct this conditional by using the strict equality operator in the conditional, whereas right now it's simply using a regular equality operator. You may recall that JavaScript has two different equality operators (as do a lot of languages): the regular equality operator (==) and the strict equality operator (===). The difference between the two is that the first equality operator doesn't force the things being compared to be the exact same (including type), whereas the strict equality operator DOES require that the things being compared are EXACTLY the same.
Thus, when the code is as follows:
//this variable is undefined, because we have not assigned anything to it
var myUndefinedVariable;
//this variable is NOT undefined, because we have assigned it a value (of "null")
var myNullVariable = null;
//uses the regular equality operator, and thus, the conditional WILL pass, because this operator doesn't check type
if(myNullVariable == myUndefinedVariable) {
identical();
}
Here, the regular equality operator is being used in the "if" conditional (i.e. the "=="), and so even though "null" and "undefined" technically aren't the same exact value, they are close enough that the regular equality operator assumes they are good enough to be considered matching, and thus the conditional passes and the identical() method is run. This is not our goal here, though, because "null" and "undefined" are NOT exactly alike. Even at just a base level, we can realize that "undefined" is a global meaning that a variable has been declared but not had anything assigned to it, whereas assigning the value of "null" to a variable is...well...assigning it a variable. Thus, something that is undefined has no assignment, and something with null DOES have an assignment. Even just there, we can see that the two variables then should NOT be considered an exact match.
Read these articles and continue to do research from here with some Google searching for further explanation:
http://blog.kevinchisholm.com/javascript/difference-between-null-and-undefined/
To suss this out, you would want to use the strict equality operator to be POSITIVE that what you were comparing was indeed identical before allowing things to proceed.
//this variable is undefined, because we have not assigned anything to it
var myUndefinedVariable;
//this variable is NOT undefined, because we have assigned it a value (of "null")
var myNullVariable = null;
//uses the strict equality operator, and thus, the conditional will NOT pass, because the values stored in the variables are NOT identical
if(myNullVariable === myUndefinedVariable) {
identical();
}
"