Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial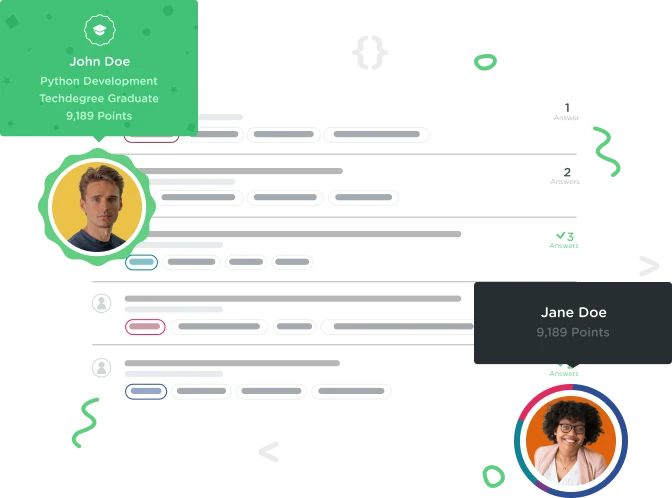
1 Answer

Maciej Sitko
16,164 PointsThe first step, is what we call a SUPER CLASS call.
It means that it utilizes parent class initializer/constructor in order to achieve and mimick some sort of classical resemblance of inheritance. What it actually does in Javascript is just mixing in parent Object to child Object.
You do this likewise:
function Dog(name) {
this.name = name;
}
Dog.prototype.bark = function() {
alert("BARK BARK BARK");
}
function Rottweiler(name, description) {
Dog.call(this, name);
this.description = description;
}
//etc, many other dogs from Dog blueprint.
NOTE: call requires you to specify context, which in this case has to be current environment this for Rottweiler.
In order to preserve a proper linkage between underlying Object.prototypes as well ( as Object itself and Object.prototype are, at this point, two different things), in the way so you can use many other prototype properties and methods of the parent, you also need to bind their prototypes in step two. Why? Because we create initializers for constructors separately as function Objects and then add properties onto the Object.prototype chain for instances.
So, you do it like this:
Rottweiler.prototype = Object.create(Dog.prototype);
This ensures that any physical instance of Rottweiler, .ie:
var R = new Rottweiler('Jelly' , 'A greate dog!');
will have access to all methods of parent and a child class and their prototype chains like in true classical inheritance example.