Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial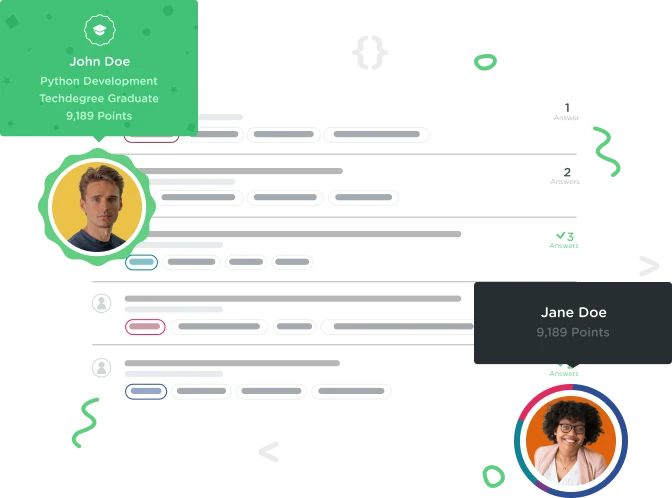

Aldo Rivadeneira
3,241 PointsIDK what's going on :/. Im not sure what's wrong with this exercise.
Im confuse with this, there's no more information about the problem i need a hand with this to understand what's wrong.
appreciate this :)
from django.db import models
# Create your models here.
class Song(models.Model):
title = models.CharField(max_length=255)
artist = models.CharField(max_length=255)
performer = models.CharField(max_length=255)
duration = models.DurationField()
def __str__(self):
return '{} by {}'.format(self.title, self.artist)
class Performer(models.Model):
name = models.CharField(max_length=255)
def __str__(self):
return str(self.name)
from django.shortcuts import render
# Create your views here.
def performer_detail(request):
return render(request, 'performer_detail.html')
def song_detail(request):
return render(request, 'song_detail.html')
def song_list(request):
return render(request, 'song_list.html')
def home(request):
return render(request, 'home.html')
{% extends 'base.html' %}
{% block title %}{{ performer }}{% endblock %}
{% block content %}
<h2>{{ performer }}</h2>
{% endblock %}
2 Answers
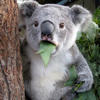
ursaminor
11,271 PointsIn your models:
-
performer
should be a foreign key in the Song class, as it refers to an object of the Performer class - no need to turn the name into a string with
str()
inPerformer.__str__()
. It's already a string.
In the views:
- you need to get the data from the db
- pass in the data to the
render()
methods
In the template:
- you need to show the songs by each performer

Aldo Rivadeneira
3,241 PointsIn the workspace i'm getting the same error, I can't found what is wrong. I'm stucked here for more than two weeks :/ @ Chris Freeman @kennethlove
from django.db import models
# Create your models here.
class Performer(models.Model):
name = models.CharField(max_length=255)
# performer = models.CharField(max_length=255, default='')
def __str__(self):
return self.name
class Song(models.Model):
title = models.CharField(max_length=255)
artist = models.CharField(max_length=255)
order = models.IntegerField(default=0)
performer = models.ForeignKey(Performer)
duration = models.IntegerField(default=0)
class Meta:
ordering = ['order',]
#duration = models.DurationField()
def __str__(self):
return '{} by {}'.format(self.title, self.artist)
# from django.http import HttpResponse
from django.shortcuts import get_object_or_404, render
from .models import Song, Performer
# Create your views here.
def song_list(request):
songs = Song.objects.all()
return render(request, 'songs/song_list.html', {'songs': songs})
def song_detail(request, pk):
#song_detail = Song.objects.get(pk=pk)
detail = get_object_or_404(Song, pk=pk)
return render(request, 'songs/song_detail.html', {'song': detail})
def performer_detail(request, pk):
performer = get_object_or_404(Performer, pk=pk)
return render(request, 'songs/performer_detail.html', {'performer': performer})
# performer = get_object_or_404(Performer, pk=pk)
# return render(request, 'songs/performer_detail', {'performer': performer})
{% extends 'base.html' %}
{% block title %}{{ performer }}{% endblock %}
{% block content %}
<h2>{{ performer }}</h2>
{% endblock %}
<ul>
{% for song in performer.song.all %}
<li>{{ song }}</li>
{% endfor %}
</ul>
Aldo Rivadeneira
3,241 PointsAldo Rivadeneira
3,241 Points@ursaminor Thank you for your answer, unfortunately, I found that in the tests there are several syntax errors, I saw this because I write the code in my local, so I could find this. The problem is when I copy and paste the code inside the test I've got the same general error so I really don't understand what's the error. :/