Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial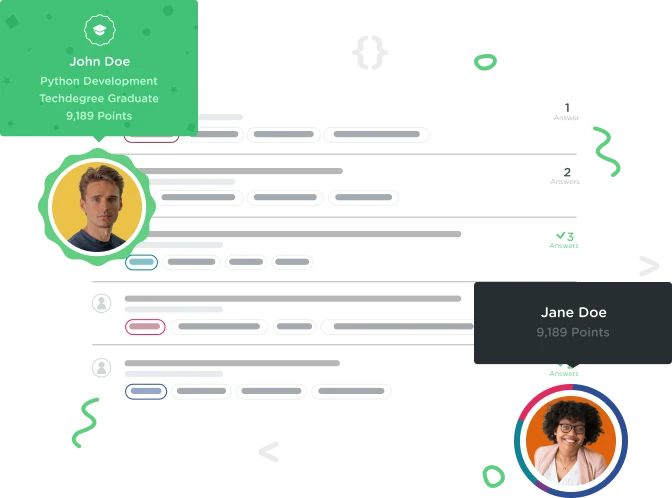

Wai Lee
13,506 PointsIf a class implements multiple protocols with initializers. How do I add all the required initializers in the same class
protocol Player { var position: Point { get set } var life: Int { get set } init(x: Int, y: Int) }
protocol Attacker { var strength: Int { get } var range: Int { get } func attack(player: Player) // Added This var name: String { get } init(name: String) }
class Enemy: Player, Attacker { var life: Int = 2 var position: Point var strength: Int = 5 var range: Int = 2 var name: String required init(x: Int, y: Int) { self.position = Point(x: x, y: y) } // What to add here? }
1 Answer
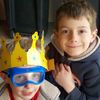
Chris Stromberg
Courses Plus Student 13,389 PointsSomething like this.
struct Point {
var x : Int
var y : Int
}
protocol Player {
var position: Point { get set }
var life: Int { get set }
init(x: Int, y: Int)
}
protocol Attacker {
var strength: Int { get }
var range: Int { get }
func attack(player: Player) // Added This
var name: String { get }
init(name: String)
}
class Enemy: Player, Attacker {
var position: Point
var life: Int
var strength: Int
var range: Int
var name: String
required init(x: Int, y: Int){
self.name = "No Name"
self.position = Point(x:x, y:y)
self.life = 10
self.strength = 4
self.range = 2
}
required init(name: String) {
self.name = name
self.position = Point(x:0, y:0)
self.life = 10
self.strength = 4
self.range = 2
}
required convenience init(x: Int, y: Int, name: String) {
self.init(x: x, y: y)
// self.position = Point(x:x, y:y)
self.life = 10
self.strength = 4
self.range = 2
self.name = name
}
func attack(player: Player){
//Do something here.
}
}
var badGuy = Enemy(x: 5, y: 6, name: "Mr. Bad")
You would then choose which initializer to use.