Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial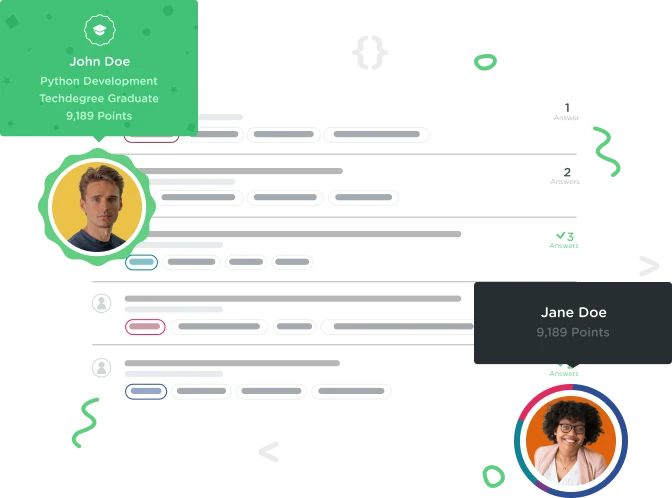

Erica DeJoannis
640 PointsIf a constant's value can't change, why does a for in loop use a constant if the value of it is going to change?
- A constant gets a value assigned.
- That value must remain the same, right?
So, wouldn't a loop want to use a variable instead of a constant?
2 Answers

Steve Smith
12,955 PointsI'm not sure what you mean here - the examples in this section use a var to store the array of items, and a for - in loop to iterate over the array, printing out each item. Where are you seeing a constant?
There are code examples in the Swift documentation like this:
let numberOfLegs = ["spider": 8, "ant": 6, "cat": 4]
for (animalName, legCount) in numberOfLegs {
println("\(animalName)s have \(legCount) legs")
}
Where a let statement is used to create a static array of values before the for - in loop iterates over them, and while this is technically correct, a static array here works, in practice arrays in applications are more typically variable, and the more common implementation of this would be var numberOfLegs = ["spider"...]
This code could be modified as follows, to use a constant inside the loop like this:
let numberOfLegs = ["spider": 8, "ant": 6, "cat": 4]
for (animalName, legCount) in numberOfLegs {
let printString = "\(animalName)s have \(legCount) legs"
println(printString)
}
Here the the output would show the same: spiders have 8 legs cats have 4 legs ants have 6 legs
This works because of the way variables are scoped - each time the for - in loop iterates, the printString variable is destroyed and created fresh, so it's not a variable being reassigned, but a constant that only exists within the scope of each individual iteration.
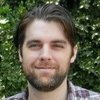
Michael Liquori
8,131 PointsI understand your confusion Erica DeJoannis, Amit does repeatedly say in the video that the x
in for x
is a constant. I think that what he means is this:
For each iteration of the array, x
has one constant value - you cannot change the value of x
inside of the for-in loop brackets. In other words, x
acts as a constant, not a variable, inside each loop iteration.
After the loop iterates one time, it increments x
, and x
remains constant at this new value for the next iteration of the loop and cannot be changed.