Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial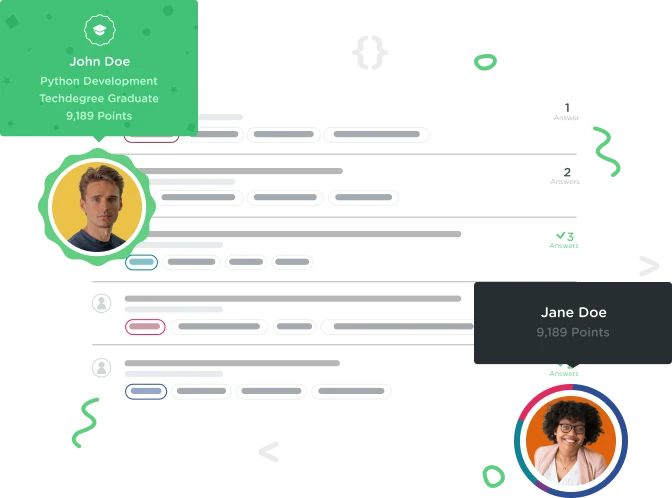

sahil jagdale
Courses Plus Student 1,043 Pointsif argument can convert in to integer , it should return int ! else the argument length * length argument ..code logic
while string == int:
is it the right way of checking the argument we inserted is int or not? missing some code logic,
1 Answer

Gavin Ralston
28,770 PointsIf you use "while string == int" what you'd be doing is checking to make sure whatever value is stored in the variable 'string' equals whatever is stored in the variable 'int' -- which could be anything at all.
It looks like he's asking you to wrap up your conversion in a try/catch block. The reason being is that you can do the following just fine:
integerOne = 1
integerTwo = "2"
return integerOne + int(integerTwo)
But you'll get a ValueError exception if you try this, because you can't convert alpha strings to numbers:
integerOne = 1
integerTwo = "Hey buddy"
return integerOne + int(integerTwo)
// everything breaks down here and Python says "ValueError: invalid literal for int()"
So in your catch statement in the challenge, where you're handling that error instead of letting your program crash, you'll want to return the result of a string multiplied by it's length.
It looks like in this challenge you're not worried about checking IF the value is an int, you're just going to TRY it, and CATCH the errors by figuring out it's not an integer when the try block failed.
Anyway, if you DO want to check the type, you could use the type() function and pass your variable in to see whether it's a string or an integer