Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial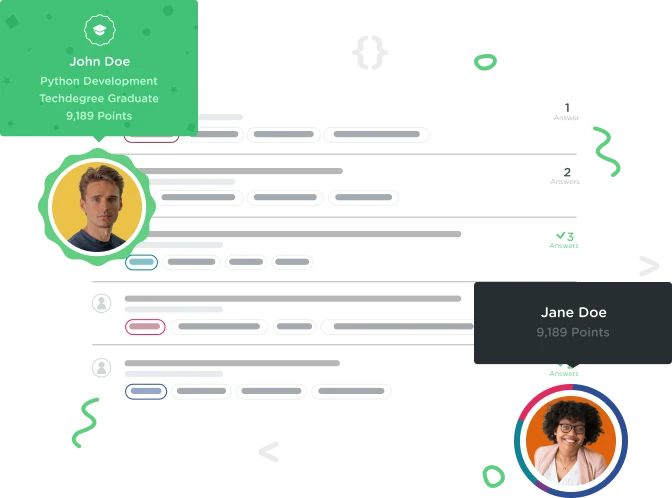

Andrew Goddard
40,807 PointsIf block resolving to false when using TryParse method
I'm trying to debug this myself, and may come to the answer without help, but thought I would ask in case anyone else hits the issue.
Everything is working in my program, all compiling fine, but I noticed the GameDate property wasn't pulling in the right date. I popped an else block in and printed to the console to confirm that if(DateTime.TryParse(values[0], out gameDate)) is resolving to false, so the value in GameDate is null, but I can't work out why.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FootballStats
{
class Program
{
static void Main(string[] args)
{
string currentDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directory = new DirectoryInfo(currentDirectory);
var fileName = Path.Combine(directory.FullName, "SoccerGameResults.csv");
var fileContents = ReadSoccerResults(fileName);
}
public static string ReadFile(string fileName)
{
using (var reader = new StreamReader(fileName))
{
return reader.ReadToEnd();
}
}
public static List<GameResult> ReadSoccerResults(string fileName)
{
var soccerResults = new List<GameResult>();
using (var reader = new StreamReader(fileName))
{
string line = "";
reader.ReadLine();
while ((line = reader.ReadLine()) != null)
{
var gameResult = new GameResult();
string[] values = line.Split(',');
DateTime gameDate;
if (DateTime.TryParse(values[0], out gameDate))
{
gameResult.GameDate = gameDate;
}
soccerResults.Add(gameResult);
}
}
return soccerResults;
}
}
}

Andrew Goddard
40,807 Pointsvalues[0] would be something like 8/26/2015 05:30 PM
I've tried looping through and just writing the value of values[0] to the console, and that worked fine, then I used the Parse method to hit the exception and it's a FormatException. The DateTime.TryParse statement doesn't like the format but I haven't worked out why yet.
2 Answers

Andrew Goddard
40,807 PointsOkay, I've sussed it out.
I'm in the UK, so my Visual Studio is configured to UK DateTime formats, and the .csv file I'm loading in has dates in the US format. I fixed it by using the overload DateTime.TryParse method that additionally takes IFormatProvider and DateTimeStyles parameters to specify that the DateTime is in the US format. All fixed, code below.
CultureInfo culture;
DateTimeStyles styles;
culture = CultureInfo.CreateSpecificCulture("en-US");
styles = DateTimeStyles.None;
DateTime gameDate;
if (DateTime.TryParse(values[0], culture, styles, out gameDate))
{
gameResult.GameDate = gameDate;
}
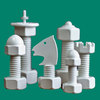
Steven Parker
231,269 PointsGood thing you found that before I got back to it, that one might have eluded me for a while!

Ariel Rzeszowski
5,482 PointsI had this same problem yersterday evening, i supposed that is need to overload but i didn't know how fill parameteres like style and Iformatprovider. Nice too see you deal with this. Thanks Steven for link as well.
CultureInfo is found in System.Globalization and i noticed that it's not about where are you, it's about what language you set on VS
Steven Parker
231,269 PointsSteven Parker
231,269 PointsWhat's in values[0]? And can you make a workspace snapshot and share the link to that?