Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial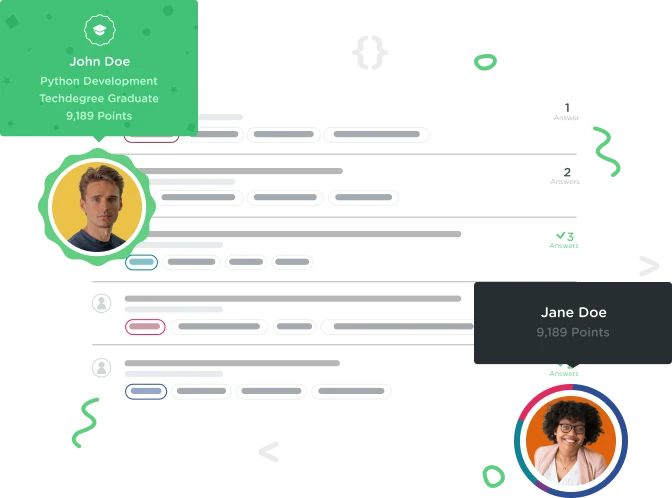

Pavel Piskunov
2,410 Pointsif else implementing in Java
Hello everybody! I`m really sorry for my english. Could anybody tell me how know Java about variable, that we declare in next line of code? For example: 1 public myCheckMethod() { 2 if (myVar == 0) { 3 return true 4 } 5 } 6 public int myVar = 0;
We check our variable on second line of code, but declare that variable only on sixth line. How Java can check value of variable, that not declared before checking?
Thank you!
1 Answer
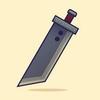
Allan Clark
10,810 PointsSo assuming your code would look like this:
//need the return type unless it is a constructor method
public boolean myCheckMethod() {
if (myVar == 0) {
return true
} else {
// all paths must return something
return false
}
}
public int myVar = 0;
This should work fine if these are both part of the same class, being that you have them both declared as public each would be a member method/variable for the class. In which case you would still want to have the member variable before the method by convention. This happens because when the Object is created the variable is already initialized, before control is able to execute the method.
The full code to execute this would look something like this
public class ExampleClass {
//need the return type unless it is a constructor method
public boolean myCheckMethod() {
if (myVar == 0) {
return true;
}
// all paths must return something
return false;
}
public int myVar = 0;
}
With control starting in the main method somewhere:
public class MainClass {
public static void main(String[] args) {
//instantiate ExampleClass
ExampleClass temp = new ExampleClass();
//this will return true
temp.myCheckMethod();
}
}
In the source code the variable is technically written after the method (again this is bad practice), but control declares and sets the variable when the Object is created, and then control calls the method so I'm not sure if this is what you had in mind. Hope this helps, please let me know if you need any further clarification.
Pavel Piskunov
2,410 PointsPavel Piskunov
2,410 PointsThank you)