Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial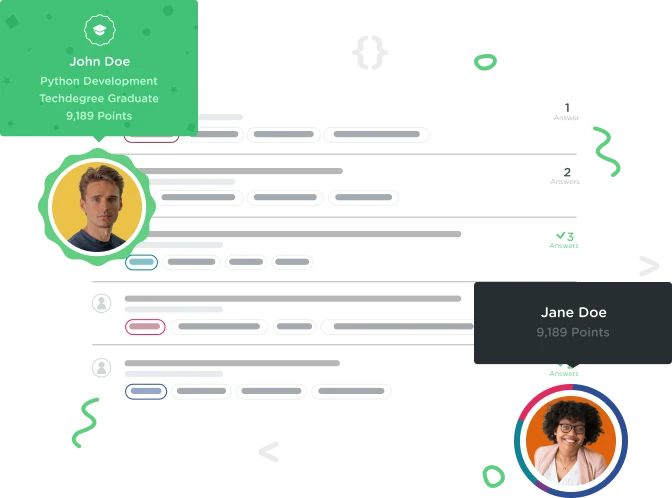
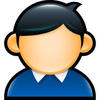
Daniel Hildreth
16,170 PointsIf Functions In JavaScript
So, I'm having trouble understanding the IF Functions in JavaScript. Can someone help explain it better to me? Like one of the issues I had was in one of the code challenges. I was asked this:
Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two.
I got this far in this code challenge:
function max( 1,2 ) { if }
I'm not sure where to go from here. I did fine in the IF function code challenges before, but for whatever reason this challenge is stumping me. You don't have to use this to try and help me understand better. But I'm getting confused on it and need some help. Thanks in advance fellow Treehouse students/teachers.
function max( 1,2 ) {
if
}
9 Answers
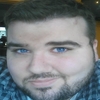
Marcus Parsons
15,719 PointsHello again Daniel,
In this exercise, you want to create a dynamic piece of code that can be used to compare any two numbers. In order to do that, you need to use variables in the function instead of static numbers such as 1 and 2. I choose to use num1
and num2
because they make sense with the function (since you're comparing two numbers), but you could name them carrot
and potato
if you wanted (although that isn't too useful lol).
So, your function should start off as:
function max (num1, num2) {
}
To setup the if statement for this challenge, take into account the logic: You want to return the larger number sent in to this function. So, one way to word your if statement is to check to see if num1
is greater than num2
and if so, return num1
. Otherwise, return num2
. You don't have to use an else here because once a function returns something, the function quits. So, we only have to put return num2
outside the if statement.
Here's all of that in action:
function max (num1, num2) {
//if the first number sent in is greater than the second
if (num1 > num2) {
//return the first number which also exits the function
return num1;
}
//if the if statement didn't go off that means that num2
//is the larger number
return num2;
}
There are actually more than a few ways to code this, but in my opinion, this is one of the simplest.

Daniel Newman
Courses Plus Student 10,715 PointsJust try to return comparison operation:
if( 1 > 2 ) return 1 if( 1 < 2 ) return 2 or if( 1 > 2 ) { return 1 } else { return 2 }
But it's "if" are not the only one case to solve this quizz.
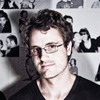
Ian Horner
22,587 PointsYou dont want to put the values 1 & 2 into the function like you have. The point of a function is that it is reusable so later down the road when you want to compare two different numbers again you dont have to write a different function.
So, where you now have 1 & 2 you need to put variables. They can be anything but it makes sense to put something simple. Let's say 'a' and then 'b'.... Or do 'Bert' and 'Ernie'
function max(a, b) {
if (a > b) {
return a
} else {
return b
}
}
So, when you call the function (below) you 'PASS' two values. max(1, 2)
'1' is where the 'a' spot is. '2' is where the b spot is in the function it sends them to the function and compares them in the if statement.
when we call it again..
max(156, 278)
it uses the same function with new numbers passed in.
here's it is with bert and ernie :)
function max(bert, ernie) {
if (bert > ernie) {
return bert
} else {
return ernie
}
}

Petar Popovic
Courses Plus Student 23,421 PointsOk Daniel, I'll try to explain.
If is conditional statement. For example you can say: if this is this then do this, or if this is that do that, and if this is not either of that two pervious things do something third.
Let's break your task to segments:
- Create a new function named max() which accepts two numbers as arguments.
What you need to write here is:
function max (numberOne, numberTwo) {
}
In this case numberOne is first argument and numberTwo is second argument
- The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which one is larger
Now you need to use if statement to say your function what to do if numberOne is larger and what to do if numberTwo is larger. You do that like this
if (numberOne > numberTwo) {
return numberOne
} else {
return numberTwo
}
What we said here is: if numberOne is larger then numberTwo return me numberOne, else return numberTwo because numberTwo is larger in that case
So your whole code should look like this
function max(numberOne, numberTwo) {
if (numberOne > numberTwo) {
return numberOne;
} else {
return numberTwo;
}
}
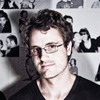
Ian Horner
22,587 PointsYou dont want to put the values 1 & 2 into the function like you have. The point of a function is that it is reusable so later down the road when you want to compare to different numbers you dont have to write a different function.
So, where you now have 1 & 2 you need to put variables. They can be anything but it makes sense to put something simple. Let's say 'a' and then 'b'.... Or do 'Bert' and 'Ernie'
function max(a, b) {
if (a > b) {
return a;
} else {
return b;
}
}
So, when you call the function (below) you 'PASS' two values.
max(1, 2)
'1' is where the 'a' spot is. '2' is where the b spot is in the function it sends them to the function and compares them in the if statement.
when we call it again..
max(156, 278)
it uses the same function with new numbers passed in.
here's it is with bert and ernie :)
function max(bert, ernie) {
if (bert > ernie) {
return bert;
} else {
return ernie;
}
}
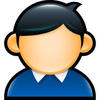
Daniel Hildreth
16,170 PointsOk thank you guys that does help explain it all in a much simpler way!
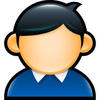
Daniel Hildreth
16,170 PointsHow would I go about calling those if functions? Could I write it like alert( num1, num2 );? Or is there a more proper way of calling it?
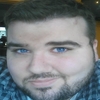
Marcus Parsons
15,719 PointsIt would be like:
alert(max(1,2));
Just wrap the alert around the function call.
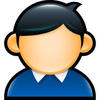
Daniel Hildreth
16,170 PointsSo would this be correct?
function max( num1, num2 ) {
if (num1>num2)
return num1;
if (num1<num2)
return num2;
}
alert( max('num1,num2') );
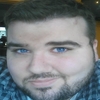
Marcus Parsons
15,719 PointsYou don't need a 2nd if statement. And you insert numbers, not strings, into the function call when you call it.
function max( num1, num2 ) {
if (num1>num2) {
return num1;
}
return num2;
}
alert( max(1,2) );
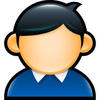
Daniel Hildreth
16,170 PointsOh ok. Thank you Marcus! I'm going to have to remember that. I keep confusing strings and numbers when calling functions.
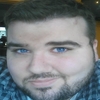
Marcus Parsons
15,719 PointsIt's okay. Just remember that if you see quotes (single or double) around values, it is a string, and if you see just a number, it's a number :P I went over why you don't need an else statement for this function in my answer, but the short and sweet of it is that once a function returns anything, the function immediately ends. So, if that if statement goes off (num1 is greater than num2) it will return num1 and stop. If that if statement doesn't execute (num2 is greater than num1), then it will return num2. That's why you don't need an else. :)