Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial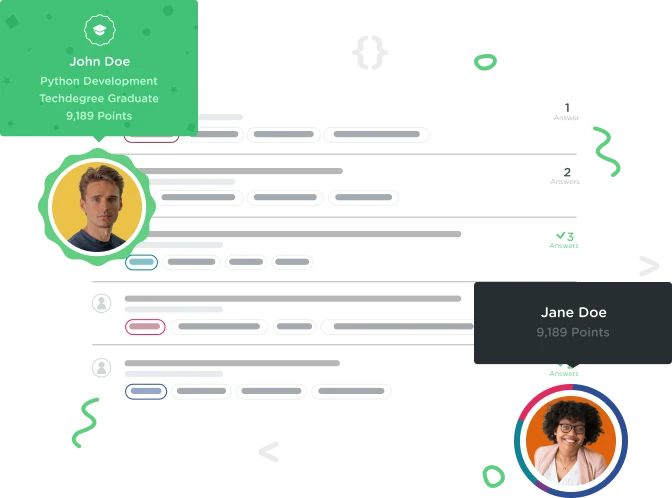

Caleb Shook
3,194 PointsIf my list consists of only vowels this will not work...
I ran it with regular words and with upper/lower case letters and it seems to work fine. If it is a word consisting of only vowels it returns what I entered.
def disemvowel(word):
word_list = list(word)
print(word_list)
vowels = ['a','e','i','o','u']
for letter in word_list:
if letter.lower() in vowels:
word_list.remove(letter)
return ''.join(word_list)
3 Answers

Cole Wilson
7,413 PointsWhen you remove the letter from word_list, you are changing the list. If you print(letter) within the inner loop before you remove it, you will see not all letters are being used.
To fix this, use a temporary variable that isn't what you loop over. You can optimize this code, but here is a working example.
def disemvowel(word):
word_list = list(word)
temp_list = list(word);
print(word_list)
vowels = ['a','e','i','o','u']
for letter in word_list:
print(letter)
if letter.lower() in vowels:
pass
temp_list.remove(letter)
return ''.join(temp_list)
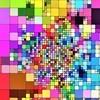
james south
Front End Web Development Techdegree Graduate 33,271 Pointsyou are modifying the list you are looping through. the remove method removes the indicated value, then shifts the remaining elements to the left. the loop moves on though, which results in elements being skipped. to fix make a copy of the list and loop through it while modifying the original, or vice-versa.

Caleb Shook
3,194 PointsThat matches up with my tests. Thank you! I was confused as to why I had to create another list but this clarified that for me.
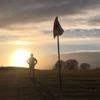
Stuart Wright
41,118 PointsRemoving items from a list while you iterate over it can lead to unexpected results. A better strategy would be to create an empty list, and as you iterate over the original word, append letters which are not vowels to the new list.