Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial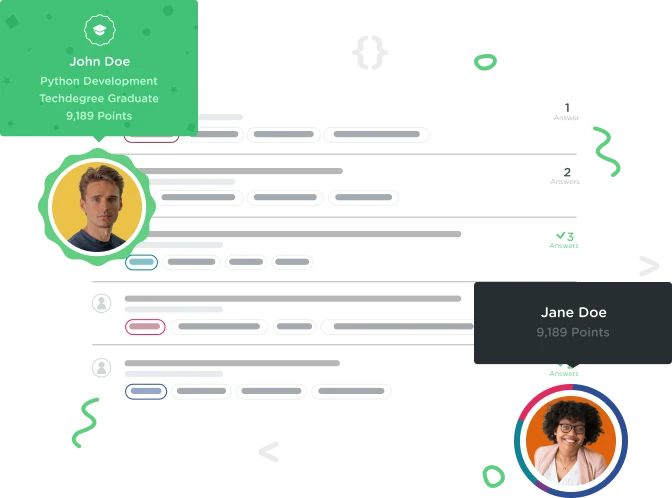

Ivan Frances Alcantara
4,528 PointsIf restaurants.copy() creates a copy, why can't we use it directly as in "for taco_joint in restaurants.copy():"?
Isn't the code creating two copies?
3 Answers

adrian miranda
13,561 PointsI'm not sure your question is clear. The following will work fine:
for taco_joint in restaurants.copy():
Perhaps you are asking, why can't you do it without the copy, like this?
for taco_joint in restaurants:
Usually, that would be fine. However, in this particular case it's a bad idea, because the code goes on to modify the list, removing certain elements. If you modify a list while you are iterating across it, the results are undefined. Think of it as trying to go up a staircase at the same time as someone else is removing some of the steps. Depending on the exact order things happen, you could hit a step just as it's removed and fall. Something similar can happen when you are modifying the list at the same time as you iterate across it.

Ivan Frances Alcantara
4,528 PointsThanks Adrian, I tried to write the code and it returned nothing, so I think I understand now the first copy is assigned to a value so that later on can be referrenced without creating more copies, and the second copy is for the for loop to work properly. Here is my try not understanding the taco_joints purpose:
all_restaurants = [ "Taco City", "Burgertown", "Tacovilla", "Hotdog station", "House of tacos", ]
def tacos_only(restaurants): for taco_joint in restaurants.copy(): if "taco" not in taco_joint.lower(): restaurants.copy().remove(taco_joint) return restaurants.copy()
dinner_options = tacos_only(all_restaurants)

adrian miranda
13,561 PointsNo, you only want to use the copy() once in this case, in the for loop.
For example, if you do:
restaurants.copy().remove(taco_joint)
That will first make a copy, then remove the taco_joint from that copy only. And at the end of the line, the copy goes away completely. You haven't modified anything that sticks around. Since your goal was to remove certain restaurants, that will not achieve your goal, because the original list hasn't been modified.
So, everything should use the original list (meaning, do NOT use copy()), except the for loop. The for loop needs to use a copy() so that the list it is using does not get changed while it is going over it. But nothing else in this function should be using a copy, because the rest of it actually wants to keep working on (and changing) the original.