Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial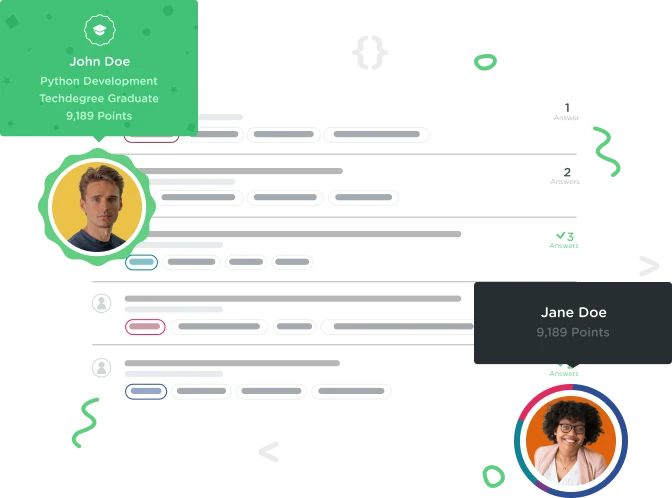

Thomas Williams
9,447 PointsIf something is an instance of a class why then cast it into that class?
I am confused as to why there is a need to cast a variable to a class type when it has already been established that the variable was already of that type. For example in the challenge it asks us to establish if obj is an instance of String, if it is, then cast it as a String!? Clearly I am missing something in my understanding of casting or instances. Any help on this would be greatly appreciated.
1 Answer
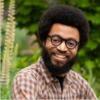
Nicolas Hampton
44,638 PointsIf you look at the method in the challenge, it says something to the effect of:
public String methodName(Object obj) { ...
this is indicating that the method accepts any Object as an argument. Everything in java is an object, it's the highest and least specific class in the language, so it doesn't really tell us much about the input going into the method. For the code we're using, we're assuming that either a string or blogpost is coming into the method, but methods can be used in different ways later, and the compiler knows that, so it won't do anything to an argument that could be anything without us promising what it can expect to get. I think this is a big reason for casting, to narrow down a vague data type of a higher class, so the compiler can know what to expect. Just because we know we'll be passing strings and blogposts to the method doesn't mean the compiler knows. It needs specifics. thus we cast the object into a more specific data type so the compiler knows what it's dealing with, like this:
String title = (String) obj;
(String) is there to let the compiler know that we can now assume obj is a String. Notice that we couldn't before, and since it could be a String or BlogPost, we need some if statements using instanceof to determine what data type obj is before we cast it appropriately. Also remember to take scope into account, as if you declare title in a scope we can't return outside of an if test, you'll get a nullpointerexception error, I believe. This is why we often declare our variables outside of the operation we initialize them, so they have a wider scope. This looks sort of like this:
String title;
if(obj instanceof String) {
title = (String) obj;
}
return title;
this allows us to manipulate the variable outside of the scope of the if statement. Also, please remember that the compiler is dumb, so initialize the variable to a default value that tells us something about what could possibly have gone wrong if we didn't get a string or a blogpost, such as:
String title = "Bad Data type";
put all these pieces together and do it for BlogPost, and you should be golden. Happy coding!
Nicolas
Thomas Williams
9,447 PointsThomas Williams
9,447 PointsHi Nicolas, I don't think my question could have been answered any better, casting now makes a lot more sense to me.
Many thanks.
Tom.
Nicolas Hampton
44,638 PointsNicolas Hampton
44,638 PointsSuper glad to hear it Tom, I hope you're having as much fun with this stuff as I am!
alexmearns
1,537 Pointsalexmearns
1,537 PointsIs casting a better option than method overloading to handle different object types?
Would the following work too?
public String getTitle(BlogPost obj) { ... }
public String getTitle(String obj) { ... }
Ray Wai
2,355 PointsRay Wai
2,355 PointsThis is a really good explanation! Way to go Mr. Hampton