Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial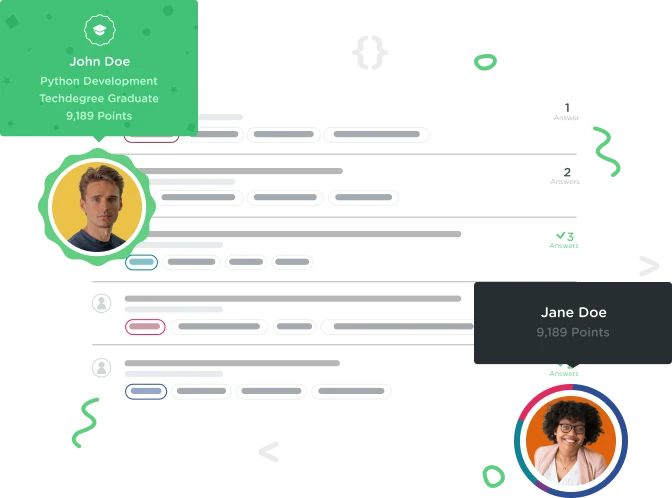

peter keves
6,854 PointsIf statement
I started to learn programming with the Swift programming course on threehouse website and I got the general idea that in if statement there is 1x if as many elseif and 1x default but since I'm learning python for quite a bit I don't quite get when we use 2x if or 2x else can somebody explaint it to me ?
1 Answer
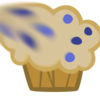
David McCarty
5,214 PointsI'm not entirely sure what you're asking but I think you mean why do we have if else and elif statements.
So, in python, if you have an if statement, you cn just leave it as an if statement with no else, or you can add an elif statement. What elif statements do is basically, in the if statemen chain, it checks if something is true or false after the if or previous elif statement, f any of the if or elif statements are true, the whole chain exits, nothing else gets checksed. E.g. say we have a few if and elif statements:
if x == 1:
# Do Stuff
elif x == 2:
# Do Stuff
elif x == 3:
# Do Stuff
elif x == 4:
# Do Stuff
else:
# Do Stuff
print("Done!")
In this instance, each elif is linked to the first if statement. If x is equal to 3, it will go through the first if and the first elif and reach the second elif. Once it tests true, it exits out of the chain and prints "Done!"
If x == 1 then the chain exits after the first if statement and prints "Done!"
If x doesn't equal any of the given statements, it defaults to the "else:" statement, which basically means, "if none of the above are true, do something"
you can also just have a bunch of if statements like so:
if x == 1:
# Do Stuff
if x == 2:
# Do Stuff
if x == 3:
# Do Stuff
else:
# Do Stuff
print("Done!")
In this instance, x is tested 3 times at each if statement. Even if one of the if statements is true, the code in that if statement is executed, but then we continue on down to the next if statement an so on; however, that last else statement will only be executed if the last if statement is false, because it's only linked to that last if statement. If we wanted all of those statements to have an else statement we would have to write one under each of them.
Essentially, elif statements have to be linked to either an if statement, or another elif statement that ends up linking to an if statement. If you have an else statement that is linked to an elif statement, that else statement will be the default for each elif statement that is linked to the same if statement.
if x == 1:
# Do Stuff
elif x == 2: # Linked to the above if statement
# Do Stuff
elif x == 3: # Linked to the above elif statement which is linked to the if statement
# Do Stuff
elif x == 4: # Linked to the above elif statement, linked to another elif statement, which is linked to the if statement.
# Do Stuff
else: # The else statement for each elif and the if statement that the elif statements are linked to
# Do Stuff
if x == 5 # Not linked to any of the above statements, it's a new if statement
# Do Stuff
else: # linked only to the last if statement. So not linked to any of those elif statements above
# Do Stuff
Not sure if that answers your question, but I hope it helped!