Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial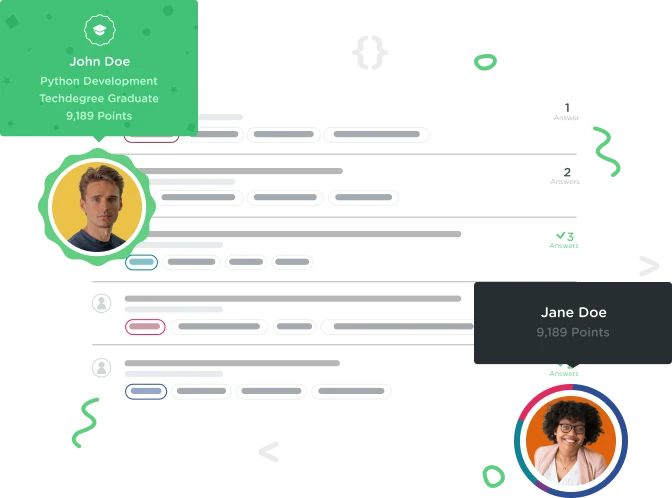
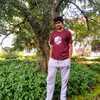
Kailash Seshadri
3,087 Pointsif statement after try statement
Instead of doing it the way Craig did it in the video, I put the if statement after the try statement, under the else. So i go something like this:
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
print ('There are {} tickets remaining'.format(tickets_remaining))
name = input('What is your name? ')
num_tickets = input('Hey, {}! How many tickets would you like? '.format(name))
#Expect a ValueError and handle it appropriately
try:
num_tickets = int(num_tickets)
#Raise a ValueError if the request is more than available.
except ValueError:
print('Please enter a whole number!')
else:
#####
if num_tickets > tickets_remaining:
print('Sorry, We do not have those many tickets! Try again!')
else:
#####
amount_due = num_tickets * TICKET_PRICE
print('The total due is ${}. Thank you!'.format(amount_due))
proceed = input('Would you like to proceed?(Please type Y or N): ')
if proceed.lower() == 'y':
print(f'Thank you for purchasing {num_tickets} tickets, {name}!')
#TO DO: Gather credit card information and process it
#AND note down f-strings(above) (Python 2.6 and above only)
tickets_remaining -= num_tickets
else:
print('Thank you anyways, {}!'.format(name))
print('Sorry {}, tickets are sold out! :-('.format (name))
What difference does it make if I do it this way, unlike the way Craig does it?
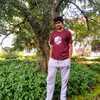
Kailash Seshadri
3,087 PointsSide note: This is what Craig did in the video, for those who have not, or don't want to , watch it:
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
print ('There are {} tickets remaining'.format(tickets_remaining))
name = input('What is your name? ')
num_tickets = input('Hey, {}! How many tickets would you like? '.format(name))
#Expect a ValueError and handle it appropriately
try:
num_tickets = int(num_tickets)
#Raise a ValueError if the request is more than available.
#####
if num_tickets > tickets_remaining:
raise ValueError ('There are only {} tickets reamining'.format (tickets_remaining))
except ValueError as err:
print('Oh no! we ran into an issue! {}. Please try again.'.format(err))
#####
else:
amount_due = num_tickets * TICKET_PRICE
print('The total due is ${}. Thank you!'.format(amount_due))
proceed = input('Would you like to proceed?(Please type Y or N): ')
if proceed.lower() == 'y':
print(f'Thank you for purchasing {num_tickets} tickets, {name}!')
#TO DO: Gather credit card information and process it
#AND note down f-strings(above) (Python 2.6 and above only)
tickets_remaining -= num_tickets
else:
print('Thank you anyways, {}!'.format(name))
print('Sorry {}, tickets are sold out! :-('.format (name))
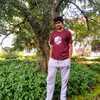
Kailash Seshadri
3,087 PointsSteven - Yeah, I'm sorry, I meant the try statement, not a for...
1 Answer
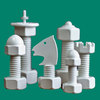
Steven Parker
241,809 PointsI assume you meant "after the try statement", in which case the difference is how a value that exceeds the number of remaining tickets will be handled. In Craig's code, the "except" is written to be generic and it handles that because of the "raise". But in your code, you handle it with a "print".
Then, you need another "else" (and a lot more indenting) to avoid dropping into the purchase code. But Craig's solution doesn't need anything extra because the exception also takes care of that.
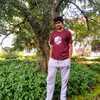
Kailash Seshadri
3,087 PointsSo there is no real difference between the works of my code and Craig's, except that his is cleaner? And yeah, I meant the try statement, not for... Sorry!
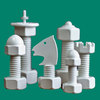
Steven Parker
241,809 PointsThe code is less compact, and the error messages are different. But I expect you intended that last difference.
Steven Parker
241,809 PointsSteven Parker
241,809 PointsI'm not seeing a "for" statement, which line is it on?