Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial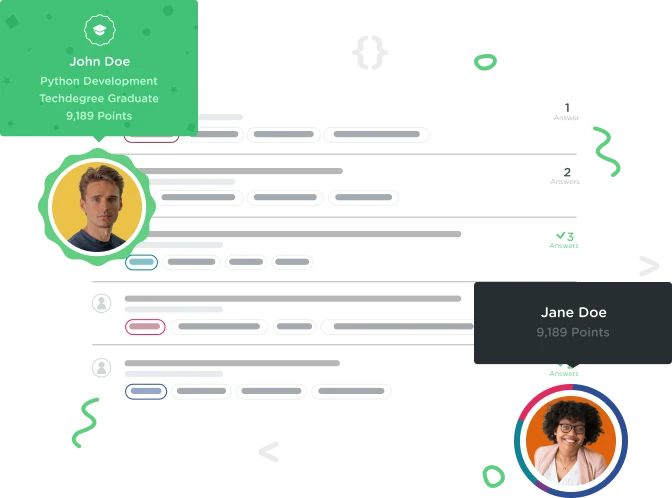
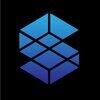
Shavaune Shackleford
9,161 Points"If that random number is even (use even_odd to find out)" how do I check if that number is even?
"If that random number is even (use even_odd to find out)"
This challenge is asking me to use a predefined function to check if the random int is even or not using an if statement. Right now I currently wrote "If even_odd(random_num)" and I don't know where to go from there because I don't know what to compare it to? The main problem is that predefined function. It only returns "not num % 2" which i don't know exactly what that means and where to go using that.
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start = True:
random_num = random.int(1, 99)
if even_odd(random_num)
3 Answers
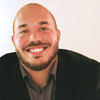
Miles Aylward
13,225 PointsWhen you use that operator, '%', it return the remainder or two divided numbers so if I divided 6%2 i would get back 0 because there is no remainder but 7%2 would give me 1. the reason it returns not is because 0 is considered false. And all other numbers are true. Meaning 6%2 would return false but in reality we need the opposite so by returning NOT NUM % 2 all even numbers will return true and odd numbers, those with remainders, will return false.
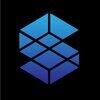
Shavaune Shackleford
9,161 Pointssolved using:
import random
start = 5 def even_odd(num): if num % 2 == 0: return True else: return False
while start > 0: random_num = random.randint(1, 99)
if even_odd(random_num) == True:
print("{} is even".format(random_num))
else:
print("{} is odd".format(random_num))
start -= 1
...... Felt like doing it this way was a bail out. I'm looking back to see if I can solve it using the original even_odd function they provided.

Daniel Schmidt
9,780 PointsTry this:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
number = random.randint(1, 99)
if even_odd(number):
print("{} is even".format(number))
else:
print("{} is odd".format(number))
start -= 1