Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial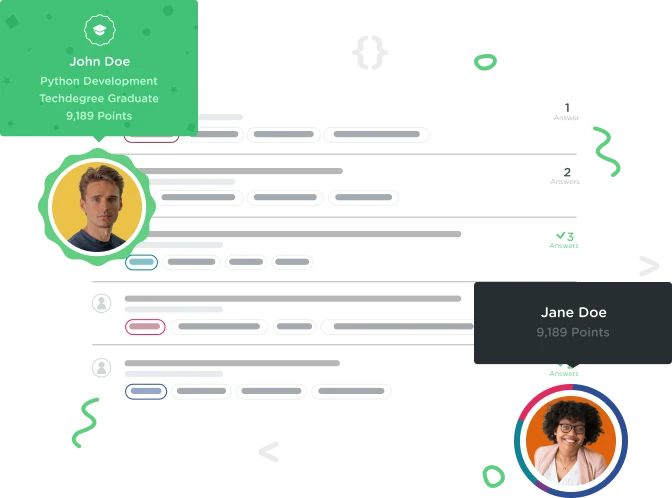
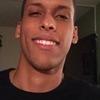
Christian A. Castro
30,501 PointsIf the argument can be converted into an integer, convert it and return the square of the number.Am I missing something?
Am I missing something out in my code?
def squared(x):
try:
if x == int(x):
return (x * x)
except ValueError:
return (x * len(x))
def squared(x):
try:
if x == int(x):
return (x * x)
except ValueError:
return (x * len(x))
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
2 Answers

Greg Kaleka
39,021 PointsSo this will fail if you pass in a "stringed" number. Let's see what happens if we run squared("10"):
def squared(x):
try:
if x == int(x): # "10" doesn't equal 10, so this won't be executed
return (x * x)
except ValueError: # No value error, though, so this doesn't either
return (x * len(x))
Nothing happens! Instead, don't bother with an if check (you're just checking if int is a number already), and just go boldly to int
ing your input inside the try block. If it's a "stringed" number, it will work, if it's already a number, it'll work, and if it's a string like "hello", it will raise a value error. You'll catch that and return the right string.
I'll let you take it from here. Lose the if statement, fix the indentation, and don't forget to int(x) both x's in the try block.
Post your solution, or let me know if you still have trouble!
Cheers
-Greg
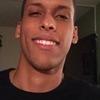
Christian A. Castro
30,501 PointsGreg Kaleka thank you so much for your quick response!! At first I didn't catch the whole point, but later it makes sense to me.. thank you so much!!
def squared(x):
try:
return int(x) * int(x)
except:
return (x * len(x))
- Cheers, Christian.