Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial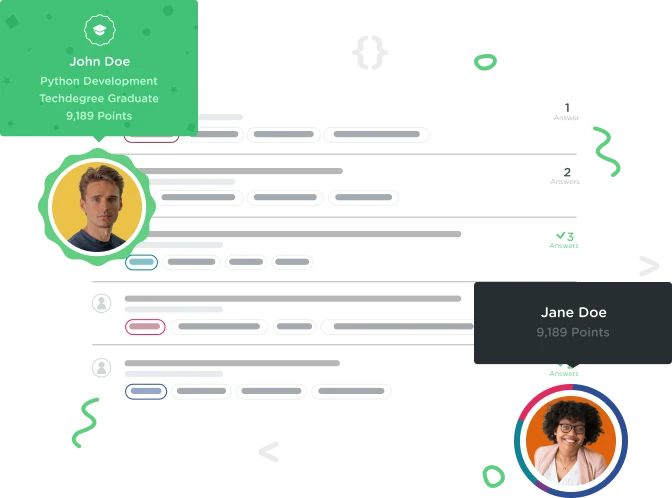
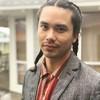
Jay Reyes
Python Web Development Techdegree Student 15,937 PointsIf the numbers are in a string, how can the route know if it's a float or int?
The below is my answer:
@app.route('/add/<num1>/<num2>')
def add(num1, num2):
# if num1 or num2 contains a . convert to float
if "." in num1 or "." in num2:
return "{} + {} = {}".format(num1, num2, float(num1)+float(num2))
else:
return "{} + {} = {}".format(num1, num2, int(num1)+int(num2))
Because I thought I can only convert once in the URL.
Back to Kenneth's answer with the 4 routes: What "built-in method" does the route have that it can recognize the parameters in the string to be floats or ints?
1 Answer
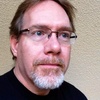
Chris Freeman
Treehouse Moderator 68,460 PointsFrom the Flask documentation, URL routes can contain
"Variable parts in the route can be specified with angular brackets (/user/<username>
). By default a variable part in the URL accepts any string without a slash however a different converter can be specified as well by using <converter:name>
."
"Variable parts are passed to the view function as keyword arguments."
The following converters are available:
string accepts any text without a slash (the default)
int accepts integers
float like int but for floating point values
path like the default but also accepts slashes
any matches one of the items provided
uuid accepts UUID strings
Multiple route (stacked decorators, in this case) may be defined for a view function.
@app.route('/add/<num1>/<num2>')
@app.route('/add/<int:num1>/<num2>')
@app.route('/add/<num1>/<int:num2>')
def view_function(num1, num2):
# process num1, num2
Post back if you need more help! Good luck1!
Jay Reyes
Python Web Development Techdegree Student 15,937 PointsJay Reyes
Python Web Development Techdegree Student 15,937 PointsDoes my browser parse the variables before the decorator recognize it with the converters?
So when I type in add/1.2/3....
My browser's request holds information that num1 is a float and num2 is an int. Then the stacked decorators are reviewed by Flask to match the request.
Chris Freeman
Treehouse Moderator 68,460 PointsChris Freeman
Treehouse Moderator 68,460 PointsThe browser sending the URL knows only text it sent. It’s the flask server doing all the interpretations of the incoming URL string and trying to massage it into one of the acceptable formats.