Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial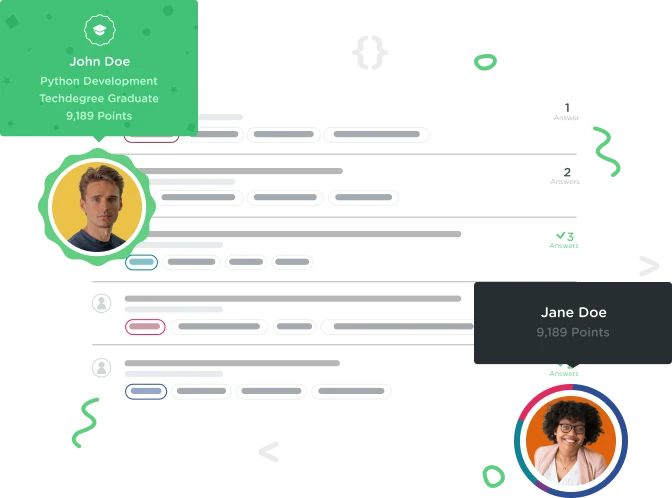
Graham Mackenzie
2,747 PointsIf we import everything from peewee, why don't we just import peewee
instead of from peewee import *
?
Looking forward to hearing the answer.
Thanks! Graham
1 Answer
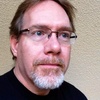
Chris Freeman
Treehouse Moderator 68,441 PointsYou absolution could simply use import peewee
. But then everywhere you use one of the fields or other peewee defined objects, you would have to prefix it with "peewee." and your code would look like:
class User(UserMixin, peewee.Model):
"""User Model
"""
email = peewee.CharField(unique=True)
password = peewee.CharField(max_length=100)
joined_at = peewee.DateTimeField(default=datetime.datetime.now)
is_admin = peewee.BooleanField(default=False)
Alternatively, you could import each peewee object individually, as needed:
# from peewee import *
from peewee import SqliteDatabase, Model
from peewee import CharField, DateTimeField, BooleanField, TextField, ForeignKeyField
from peewee import IntegrityError
Graham Mackenzie
2,747 PointsGraham Mackenzie
2,747 PointsThanks again, Chris!
But your answer makes me think of another question: In that case, why don't we import datetime thusly
from datetime import *
so that we can drop appendingdatetime
to the front of all related functions? : )Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsFirst, understand that the
from peewee import *
is extremely bad form, but due to legacy implementation it is accepted as "tolerable".The biggest reason to not use
import *
is namespace. By using the star method, everything from the other module is placed into the main namespace. It is very possible that something in your code would collide with something from another module or could collide between two modules if both were imported using the star.The other risk is future changes. Say your code works with the
import *
, but then the other module updates and add new objects that then collides with your code. Your code breaks without changes from you.For
datetime
, it is common to seefrom datetime import datetime
so one prefixed "datetime." can be dropped.Graham Mackenzie
2,747 PointsGraham Mackenzie
2,747 PointsThanks again!
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsAs a closing comment, the direct imports from peewee I show in my answer are the actual imports I used to solve the Build a Social Network with Flask Tacocat challenge. I wanted to see what imports were required if the import star wasn't used.