Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial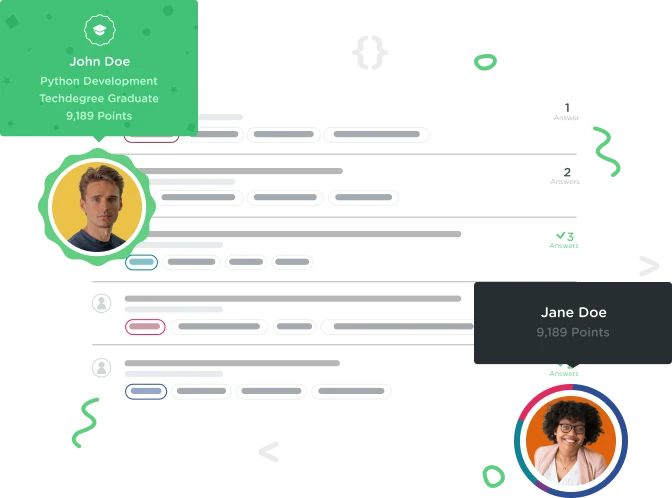

Gracie Jensen
1,942 PointsIf you change an attribute for one instance, how do you get it to also change when you edit the entire class?
I understand that we've edited the .doors
of car_one to be 6 while the Car
class still has doors = 4
, it's now stored somewhere else on the computer's memory and won't be edited with the rest of the class. I then went in and manually changed car_one.doors = 2
and it showed that it was now stored on the same location as car_two and the Car class. But then when I changed Car.doors = 4
, car_one didn't update. Is there a reason it didn't change even though at one point it was again stored on the same location? And, probably more importantly, is there a way to re-group it or whatever?
class Car:
wheels = 4
doors = 2
engine = True
car_one = Car()
car_two = Car()
car_one.doors = 6
id(car_one.doors) #139921268173264
id(car_two.doors) #139921268173168
id(Car.doors) #139921268173168
car_one.doors = 2
id(car_one.doors) #139921268173168
id(car_two.doors) #139921268173168
id(Car.doors) #139921268173168
Car.doors = 4
car_one.doors #6
car_two.doors #4
Car.doors #4
1 Answer
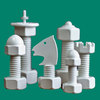
Steven Parker
231,599 PointsAn instance variable will take precedence over a class variable of the same name. So initially, when you create the two instances, they only have the class variable "doors" which they share.
But when you assign car_one.doors, that creates a new instance variable for car_one but it has no effect on car_two. When you later check the value of car_one.doors you will see the instance variable instead of the class variable.
But when you check the value of car_two.doors, you still see the value of the class variable.
If you want car_one to go back to using the shared class variable, you can delete the instance variable.
car_one.doors = 2 # creates instance variable unique to car_one
Car.doors = 4 # updates the shared class variable
print(car_one.doors) # 2 (instance variable)
print(car_two.doors) # 4 (class variable)
print(Car.doors) # 4 (class variable)
del car_one.doors # deletes the instance variable
print(car_one.doors) # 4 (class variable)