Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial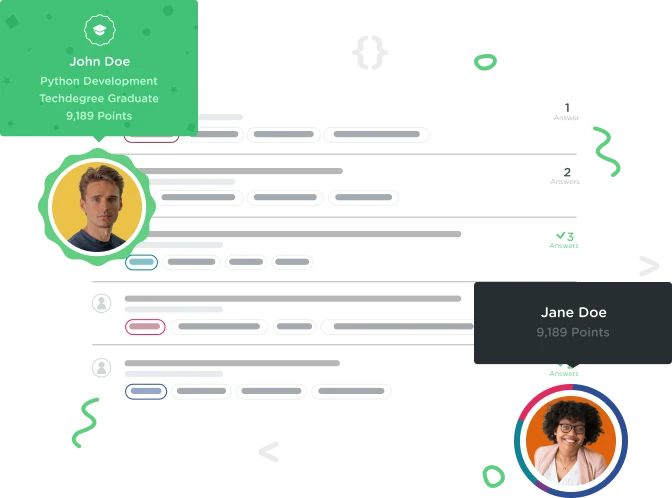

Alan Temirov
Courses Plus Student 762 PointsIf/Elif
Is there a way where I can add several names to if and elif,so it would be like elif/if first_name = "Alex" … and lets say 2 other names, how can plunge them in the same line,cause typical for now commas and + sign don't seem to add them.
4 Answers
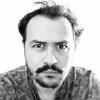
ANTOINE CREIS
1,596 PointsHi Alan, Not sure I understood your question, but here is something that might help you. You should use "or" in your function to add more than one name and not "+" So your code line should look like this:
elif first_name == "Alan" or "Antoine" or "Chris" :
print... rest of your code
hopefully this helps !
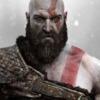
boi
14,242 PointsHey there Alan!
I am not sure what you mean by your question but check this out;
first_name = "Alan"
last_name = "Temirov"
full_name = "Alan Temirov"
if first_name == "Alan" and last_name == "Temirov" or full_name == "Alan Temirov":
print("Present")
else:
print("Absent")
Is this what you're looking for?

Alan Temirov
Courses Plus Student 762 PointsThanks for the answer!But I was talking about how do I put more than 1 First names in the elif,when I type 3 names(not one as it was in the video) in the input line,it triggers the elifso it looks like this: elif first_name == "first_nameX" +"first_nameY" +"first_nameZ",but the + sign doesnt seem to do that,not does the comma,thats why Im confusesld
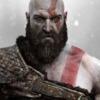
boi
14,242 PointsWhat you describe is logically correct but won't give you the answer you might be expecting, for example;
my_name = "AlanTemirov"
first_name = "Alan"
last_name = "Temirov"
if my_name == first_name + last_name:
print("Yes that's my name")
else:
print("Not my name")
The above code is based on your logic implementation.
If you want to add multiple conditions you could do something like this;
name = "Alan"
first_name = "Alan"
middle_name = "Alan"
last_name = "Alan"
full_name = "Alan Temirov"
no_name = "Nil"
if name == first_name and name == middle_name and name == no_name:
print("{}".format(full_name))
elif name == first_name and name == middle_name and name == last_name and name == full_name:
print("My name is {},{},{}".format(first_name,middle_name,last_name))
elif name == first_name and name == middle_name and name == last_name or name == full_name:
print("My name is {} {} {} {}".format(first_name,middle_name,last_name,full_name))
But this is a wacky way to do it, instead, you could use a function or a for loop
If you don't understand the logic of the operators "and", "or", let me know.
Also when you are writing code make sure you format them into "python syntax".
Click Here to learn how to use Markdown.
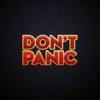
Hambone F
3,581 PointsSome already suggested the straightforward approach:
if first_name == "Alex" or first_name == "Bob" or first_name == "Alice":
print(first_name, "is one of the names I'm checking for!")
However, you can also check if the name is in a list of names - to me, this is easier to read:
if first_name in ["Alex","Bob","Alice"]:
print(first_name, "is one of the names I'm checking for!")
You could even make that a variable defined earlier or elsewhere:
# Easier to add new names this way
names_to_check = ["Alex", "Bob", "Alice"]
if first_name in names_to_check:
print(first_name, "is one of the names I'm checking for!")
Alan Temirov
Courses Plus Student 762 PointsAlan Temirov
Courses Plus Student 762 PointsYeah,that what I was looking for, I use "or"! Thank you!
Hambone F
3,581 PointsHambone F
3,581 PointsHi Antoine,
Just a quick note here - while that statement will not throw an error, it does contain a logical error.
I think you meant:
As written, the check is actually like this:
(first_name == "Alan") or ("Antoine") or ("Chris")
Which will always evaluate to True. Well, technically, unless the persons name is Alan, that operation will always return "Antoine", which is 'truthy'.
Try it in the terminal and see!