Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial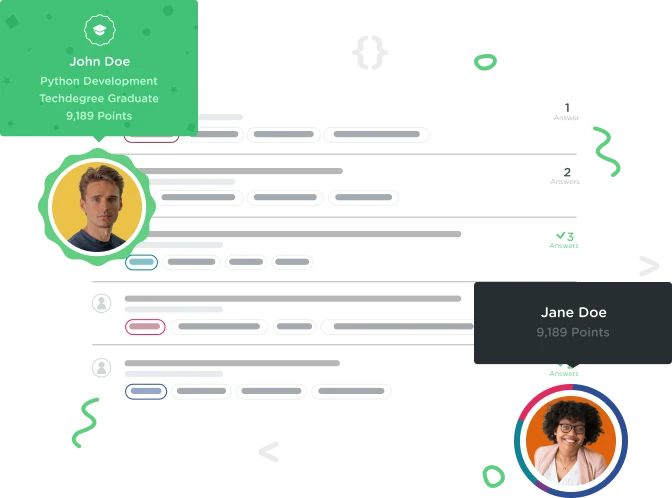

Hamzah Iqbal
Full Stack JavaScript Techdegree Student 11,145 PointsIf/else if is driving me nuts.
I've been trying solve this problem for some time. I've also looked into other members' discussions with no result.
using System;
class Program
{
static string CheckSpeed(double speed)
{
if (speed > 65)
return string ("too fast");
}
{
else if (speed < 45)
return string ("too slow");
}
{
else ("speed OK");
}
static void Main(string[] args)
{
Console.WriteLine(CheckSpeed(44));
Console.WriteLine(CheckSpeed(88));
Console.WriteLine(CheckSpeed(55));
}
}
1 Answer
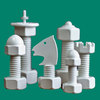
Steven Parker
231,275 PointsYou have the right idea, but a few syntax issues:
- the function body should be just one block of code between braces
- braces around conditional code blocks begin after the conditional expression
- braces around conditional code blocks are optional if the block has just one statement
- "string" is not a function, and literals don't need type declarations
- there is a "return" missing between the final else and the string
- strings don't need to be enclosed in parentheses
static string CheckSpeed(double speed)
{
if (speed > 65)
return "too fast";
else if (speed < 45)
return "too slow";
else
return "speed OK";
}