Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial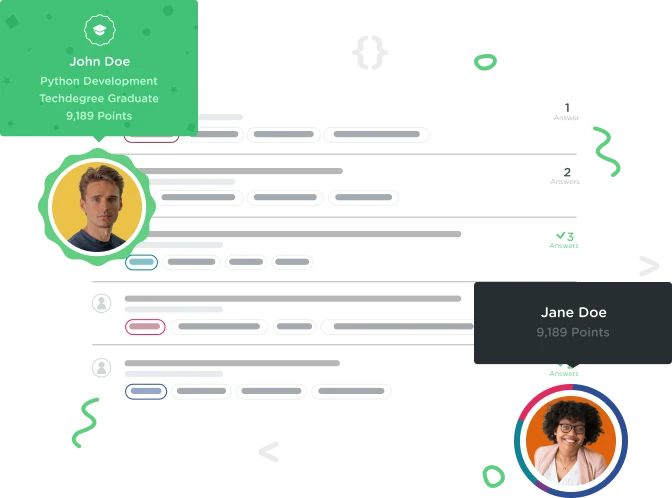

Julian Betancourt
11,466 PointsIf/else statements and concatenation question
Here's the code I'm using for this challenge :
var verb = prompt("Please type a verb");
var adjective = prompt("Please type an adjective");
var noun = prompt("Please type a noun");
var noun2 = prompt("Please type another noun");
alert("Are you ready?")
var finalSentence = "<p>Once upon a time there was a ";
finalSentence += noun + " that liked to ";
finalSentence += verb + " the ";
finalSentence += adjective + " " + noun2 + "</p>";
document.write(finalSentence);
I realized that if the user uses a noun that starts with a vowel, then the sentence is grammatically incorrect:
e.g
Once upon a time there was a [arm] that liked to [see] the [great] [girl].
So, how do I code that if the noun following an "a" starts with a vowel or h-vocal, then replace that "a" with "an".
1 Answer
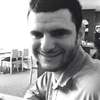
Dan Garrison
22,457 PointsHi Julian,
I would probably recommend using function with a for loop and an if statement. Check out my code below and see if you can figure out what I did before I explain it. Please note that you will need to modify the code slightly for your purposes, but the general idea should be the same.
function myAdjective() {
var vowels = ['a', 'e', 'i', 'o', 'u']
var input = prompt('Please type an adjective');
for (var i = 0; i < vowels.length; i++) {
if (input[0] === vowels[i]){
return "an " + input
}
}
return "a " + input
};
Since the situation you described usually occurs when the next word begins with a vowel we need to a create a list with all of the vowels in it. Next we prompt the user to type in the word. We use the for loop to loop through the vowels list. Next, we use the if statement to compare the first letter of the input (that's what that 0 in brackets does) with each of the vowels in the vowels list. If any of them match, it breaks out of the loop returns "an " + input. If it does not find a match then it breaks out of the for loop and just returns "a " + input.
Here is my complete code so you can have an idea on how to integrate the function:
var adjective = myAdjective()
var verb = prompt('Please type a verb');
var noun = prompt('Please type a noun');
alert('All done. Ready for the message?');
var sentence = "<h2>There once was " + adjective;
sentence += ' programmer who wanted to use JavaScript to ' + verb;
sentence += ' the ' + noun + '.</h2>';
document.write(sentence);
function myAdjective() {
var vowels = ['a', 'e', 'i', 'o', 'u']
var input = prompt('Please type an adjective');
for (var i = 0; i < vowels.length; i++) {
if (input[0] === vowels[i]){
return "an " + input
}
}
return "a " + input
};