Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial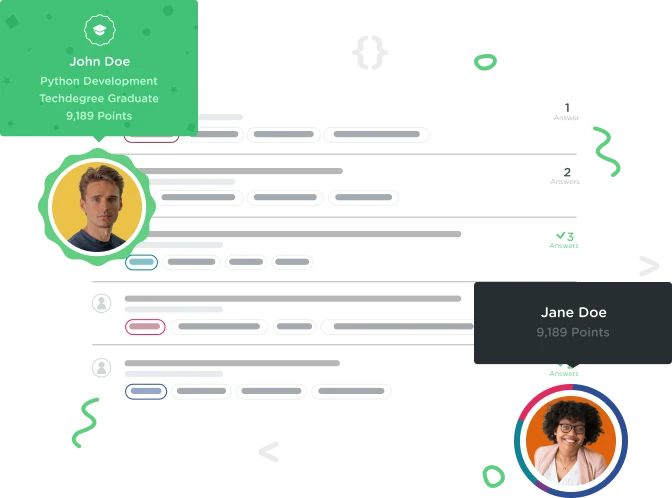

silashunter
Courses Plus Student 162 PointsIfs instead of Elifs
I used just all IFs. No ELIFs. Still works !
What is point of using ELIFs ?
P.s.: I have somehow ( I didn't know it is possible ) fast forwarded playback video of this lecture, but can't turn it back ! Please help.
shList = []
def show():
print('Write things into your shopping list !')
print('When you are done, write DONE !')
print('When need help, write HELP !')
print('When need to see list, write SHOW !')
def write():
while True:
inpSaved = input('> ')
if inpSaved == 'DONE':
break
if inpSaved == 'HELP':
show()
continue
if inpSaved == 'SHOW':
full()
continue
shList.append(inpSaved)
print('Added ! List has', len(shList), 'items.')
def full():
print('Here are your items: ')
for item in shList:
print(item)
show()
write()
full()
1 Answer
William Li
Courses Plus Student 26,868 PointsWell, in the code you've written, there's no difference in using multiple if VS elif, because the conditions you're check for can only be one Truth.
But there's important distinction. Using elif, if one of the condition is met, it will stop and won't continue checking the rest of the elif chains; whereas using multiple if, it will always check all the conditions regardless.
def mul1(num):
if num%3 == 0 && num%5==0:
print("multiples of 3 & 5")
if num%3 == 0:
print("multiples of 3")
if num%5 == 0:
print("multiples of 5")
def mul2(num):
if num%3 == 0 && num%5==0:
print("multiples of 3 & 5")
elif num%3 == 0:
print("multiples of 3")
elif num%5 == 0:
print("multiples of 5")
mul1(15)
# => multiples of 3 & 5
# => multiples of 3
# => multiples of 5
mul2(15)
# => multiples of 3 & 5