Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial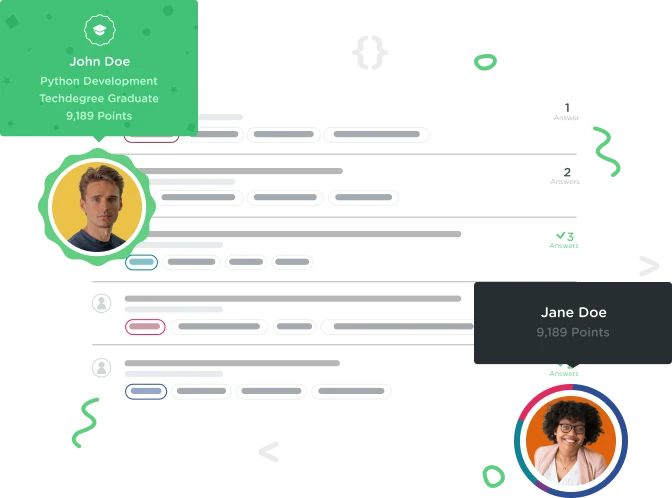

Anthony De Smet
9,666 PointsIllegalArgumentException
I can't find what is wrong, it says that I didn't cath the exception
this is my code:
public class Main { public static void main(String[] args) { GoKart kart = new GoKart("yellow"); if (kart.isBatteryEmpty()) { System.out.println("The battery is empty"); } kart.drive(2); try { kart.drive(100); System.out.println("This will never happen"); } catch (IllegalArgumentException iae){ System.out.println("Whoa there!"); System.out.printf("The error was: %s\n", iae.getMessage()); } } }
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
kart.drive(2);
try {
kart.drive(-20);
System.out.println("This will never happen");
} catch (IllegalArgumentException iae){
System.out.println("Whoa there!");
System.out.printf("The error was: %s\n", iae.getMessage());
}
}
}
3 Answers

Marileen Mennerich
1,161 PointsYour code is pretty much fine. The only problem is that right above your try/catch block, the drive()-method is called. Since this call is outside of the try, the exception is not caught. Remove it or move it into your try block. The drive(-20) call is not necesarry in the latter case.

MUZ140265 Blessing Kabasa
4,996 Pointsfirstly there is no need to have two drive methods , drive(2) and drive(-20) Secondly, there is no need to write this statement in try, System.out.println("This will never happen");
you may use the code bellow and give me a shout if it helps
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(2);
} catch (IllegalArgumentException iae) {
System.out.println("Alert");
System.out.printf("There was an error: %s\n", iae.getMessage());
}
}
}

Jess Sanders
12,086 Pointstry {
dispenser.load(400);
} catch (IllegalArgumentException iae) {
System.out.println("Whoa there!");
System.out.printf("The error was: %s%n", iae.getMessage());
}
Above, is the code example to follow. Instead of dispenser.load(400), you are trying kart.drive(2)