Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial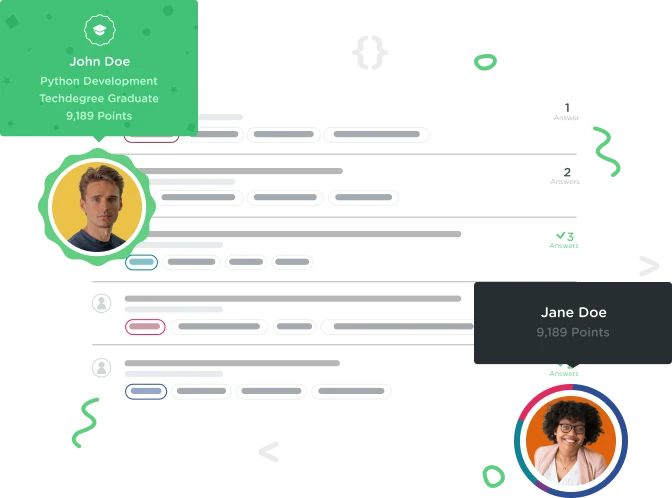

Paul Ryan
531 PointsIllegalArgumentException
Stuck on this one. Having trouble with the drive method (line 30 - 37). Trying to return the message "Not enough charge" if there's not enough bars/charge left in the battery to drive another lap. (1 lap driven equals -1 bar of charge)
I get the message "Make sure you throw a new IllegalArgumentException if the requested amount of laps would make the battery less than zero." Which I think I've already done.
If i understand correctly, I'm not telling java what to do if chargeAvailable is > 0.
Almost understanding this one completely I think so would really appreciate finding the missing link.
Thank you in advance, Paul "Accounting by day, coding by night!"
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
int chargeAvailable = MAX_BARS - lapsDriven;
if (chargeAvailable < 0) {
throw new IllegalArgumentException("Not enough charge!");
}
barCount = chargeAvailable;
}
}
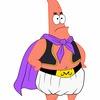
<noob />
17,062 PointsHi, You have few mistakes, The challange asks u to write code to check when your kart is out of battery, How u do it? You need to look at 2 things: first, as u see you have the "barCount" private var that represent how much battery you have left in your kart. second, you also have the "lapsDriven" private var that suppose to to represent how many laps you have driven already.
After u looked at those 2 variables ask yourself how u check when your out of battery, The answer is this, you need to check if lapsDriven is greater than barCount(each lap the kart does cost 1 battery), if it is you throw the exception.
public void drive(int laps) {
if (laps > barCount) {// in this line you check if lap is greater than barCount
throw new IllegalArgumentException("Not enough battery remains"); //then u throw an excpetion
}
lapsDriven += laps;
barCount -= laps;
}
}
i hope you understand this explantion im also kinda new to java ;D

Paul Ryan
531 PointsThank you for your post. It has worked. However if you have the time I do not fully understand one thing.
In your code you say the following:
if (laps > barCount)
then the IllegalArgumentException will run.
In my code MAX_BARS = 8. Driving 1 lap drains 1 bar. So after lap 5 there will be 3 bars left and laps will be greater than barCount. The IllegalArgumentException will now run 3 laps early. (ie we should be able to drive 8 laps not just 5)
Or is it that the integer laps is always zero?
Thank you for any further insight you can offer. It is much appreciated.
If you post an answer instead of a comment i can upvote it for you!
2 Answers
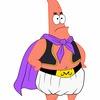
<noob />
17,062 PointsHi, You have few mistakes, The challange asks u to write code to check when your kart is out of battery, How u do it? You need to look at 2 things: first, as u see you have the "barCount" private var that represent how much battery you have left in your kart. second, you also have the "lapsDriven" private var that suppose to to represent how many laps you have driven already.
After u looked at those 2 variables ask yourself how u check when your out of battery, The answer is this, you need to check if lapsDriven is greater than barCount(each lap the kart does cost 1 battery), if it is you throw the exception.
public void drive(int laps) {
if (laps > barCount) {// in this line you check if lap is greater than barCount
throw new IllegalArgumentException("Not enough battery remains"); //then u throw an excpetion
}
lapsDriven += laps;
barCount -= laps;
}
}
i hope you understand this explantion im also kinda new to java ;D
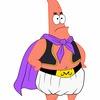
<noob />
17,062 PointsHi again!, Your only missing 1 thing to understand it :0 The IllegalArgumentException will not run in the case you presented, why not? >> because the exception will run after the variable laps will overcome(will pass) the variable barCount. for example, if you drive 9 laps then the exception will be thrown because barCount only contains 8 batteries. Another explantion that might benfit as well: if laps = 6 and battery = 2, the exception will not run because you have 2 batteries left, the excpetion only runs when u have 0 batteries.
I hope u fully understand it now :D, I will post my answer again , ill be happy if u can mark as best answer
Paul Ryan
531 PointsPaul Ryan
531 PointsI now see that you can't see the lines when I ask a question. It's the last method.