Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial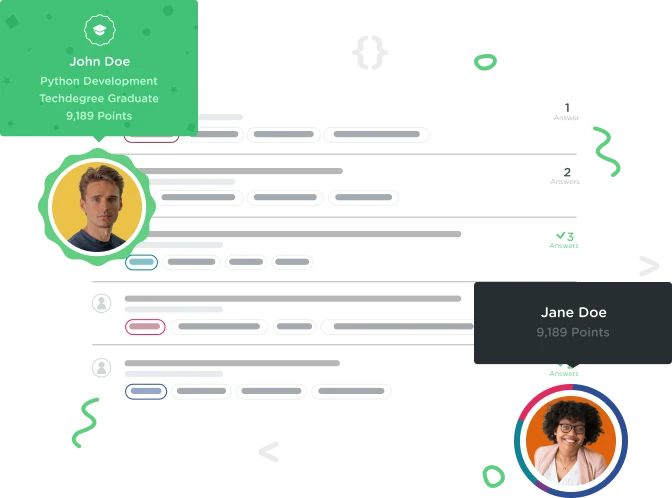
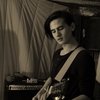
Kristinn Godfrey
6,161 PointsI'm 99% sure there's nothing wrong with my tuples solution
Hey my following code gets the error: "Bummer! Didn't get the right output. For example, expected (10, 'T') as the first item, got (10, 'T') instead."
That's the same value!
I already got it right by replacing my iteration from tuples indexes to "n" index.
Is there something wrong with doing it this way?
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# If you use .append(), you'll want to pass it a tuple of new values.
def combo(some_list, some_string):
new_list = []
for n in range(len(some_string)):
new_list.append((some_list[0],some_string[0]))
return new_list
2 Answers
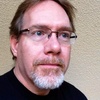
Chris Freeman
Treehouse Moderator 68,423 PointsRunning your code interactively shows the issue:
$ python3
Python 3.4.0 (default, Jun 19 2015, 14:20:21)
[GCC 4.8.2] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> def combo(some_list, some_string):
... new_list = []
... for n in range(len(some_string)):
... new_list.append((some_list[0],some_string[0]))
... return new_list
...
>>> combo([1,2,3,4,5,6], "abcdef")
[(1, 'a'), (1, 'a'), (1, 'a'), (1, 'a'), (1, 'a'), (1, 'a')]
You need change the index to n instead of the fixed 0:
... new_list.append((some_list[n],some_string[n]))
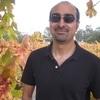
Kourosh Raeen
23,733 PointsYou could also write your loop in a different way so that you don't have to worry abut the index:
def combo(some_list, some_string):
new_list = []
for item, letter in zip(some_list, some_string):
new_list.append((item, letter))
return new_list
``
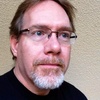
Chris Freeman
Treehouse Moderator 68,423 PointsNice! If you're going to use zip
go all the way. Since zip
returns a list
the for
loop iteration becomes redundant.
def combo(some_list, some_string):
new_list = list(zip(some_list, some_string))
return new_list
# or simply
def combo(some_list, some_string):
return list(zip(some_list, some_string))
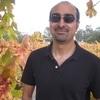
Kourosh Raeen
23,733 PointsThat's awesome! Thanks for the tip Chris Freeman !
Kristinn Godfrey
6,161 PointsKristinn Godfrey
6,161 PointsThanks, I'm on a crossroads of new information, thought 0 there wasn't a fixed size
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsThe index notation of
[0]
means the first element ("the zero-th")