Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial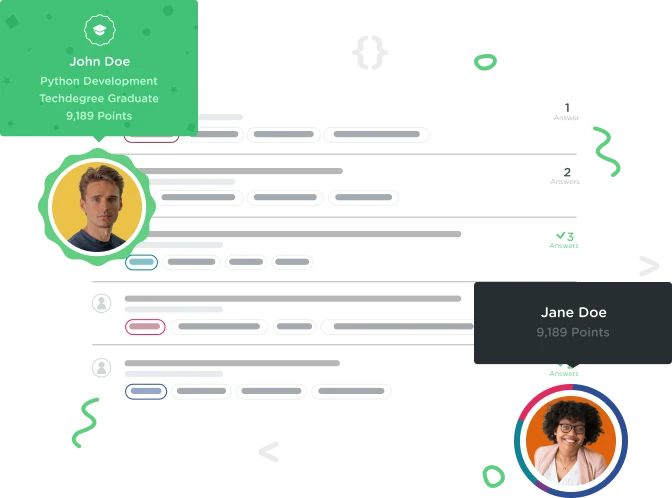

Adrian Kritzinger
Front End Web Development Techdegree Graduate 12,948 PointsI'm a little confused by the instructions and why my method of getting the correct element doesn't work.
I'm a little confused by the instructions and why my method of getting the correct element doesn't work.
In the second part of the challenge the instruction says: Next, set the text content of the <p> element with the class info to the value stored in inputValue.
So, both my brain and the instruction says I should be looking for a <p> element with a class name "info" so I typed in:
document.getElementsByClassName('info').textContent = inputValue;
The above gives me a response asking if I'm sure I'm targeting the <p> element, which I thought I was.
Eventually I used the following line of codem because it's the first and only <p> on the page, adn this worked:
document.querySelector('p').textContent = inputValue;
Why did the first option not work?
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<label for="linkText">Link Text:</label>
<input type="text" id="linkText" value="sample text">
<p class="info"></p>
</div>
<script src="app.js"></script>
</body>
</html>
2 Answers

Simon Voort
5,682 PointsYou're doing great, and your solution works well for the page you got from the challenge. However, to understand the difference between selecting a class, and an element with a class, let's imagine that you got a slightly different page, with two elements that both have the class info:
<p class="info"></p>
<div class="info"></div>
When you use document.getElementsByClassName("info"), you will get both the p and the div element.
The challenge here is very specific, and it wants you to select only p elements that also have the class info. With querySelector, you can filter more precisely, and select elements of a specific type with a specific class.
For example: document.querySelector("p.myClassName")
selects all p tags with the class myClassName, and won't select div elements.

devvoyage
Front End Web Development Techdegree Student 15,200 PointsHi there, Adrian Kritzinger! I see you've already received a pretty solid answer. However, I feel like there's something important to note here. The querySelector
always returns a single item as a "node." But getElementsByClass
is a little different. It returns an HTMLCollection
. Note that in getElementsByClass
the "Elements" part is plural. Even if there is only one match (or even zero), it returns a structure that is array-like.
So, you would need to ask for the first one returned using indexing.
You were actually very close the first time.
Instead of:
document.getElementsByClassName('info').textContent = inputValue;
You would have needed to tell it to get the first one. An HTMLCollection (much like an array) does not have a textContent
property. Rather, each item in the collection does.
document.getElementsByClassName('info')[0].textContent = inputValue;
Hope this helps!