Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial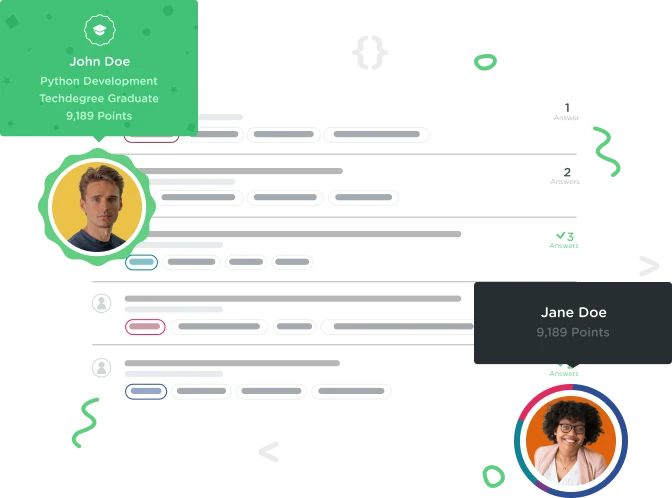

Aaron Glaesemann
11,303 PointsI'm afraid that I don't quite understand what this challenge is asking me to do.
Final challenge of Swift 2.0 enumerations
Could someone explain what I'm supposed to do in the part 2 of the challenge? I apologize but I'm fairly confused. I feel like I was progressing nicely until this challenge.
Here is the code I wrote in the enums.swift tab:
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonStyle {
switch self {
case Button.Done: return UIBarButtonStyle.Done
case Button.Edit: return UIBarButtonStyle.Plain
}
}
}
let done = Button.Done("Done")
let doneButton = done.toUIBarButtonItem()
Thanks in advance and for your explanation and time.
1 Answer
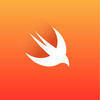
Steven Deutsch
21,046 PointsHey Aaron Glaesemann,
You're really close, there's just a few things we need to look at. This was the HARDEST code challenge for me too.
First, this is an instance method. We need the method to return an instance of the UIBarButtonItem. So, it has to have a return type of UIBarButtonItem.
Second, to create the instance, we have to pass UIBarButtonItem four arguments. You can see these properties defined on the Buttons.swift page in the UIBarButtonItem class. They are: title, style, target, and action.
Third, to get the value of title, we have to access the associated value of the Button type. We can do that by assigning it's value to a local constant in each case.
Fourth, you then return a call to create an instance of UIBarButtonItem for these two cases, with their respective arguments. You can pass nil in for the target and the action.
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem {
switch self {
case .Done(let title): return UIBarButtonItem(title: title, style: UIBarButtonStyle.Done, target: nil, action: nil)
case .Edit(let title): return UIBarButtonItem(title: title, style: UIBarButtonStyle.Plain, target: nil, action: nil)
}
}
}
let done = Button.Done("Done")
let doneButton = done.toUIBarButtonItem()
The rest of your code is correct. Good luck!
Aaron Glaesemann
11,303 PointsAaron Glaesemann
11,303 PointsThank you! It all makes sense and I just felt a huge rush of euphoria as it finally "clicked."
Moments before I read your explanation, I actually solved it with the following code:
I see now that I wasn't returning an object from the class UIBarButtonItem, I was actually returning UIBarButtonStyle. I appreciate the further explanation as it reinforced my recent realization and will help with retention. This community is awesome! Thanks again!