Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial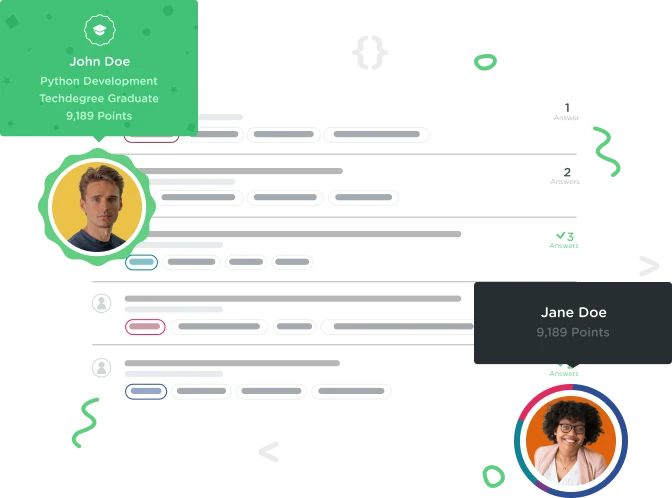

Kim Dallas
11,461 PointsI'm completely lost with this one.
how can I write a loop that repeats the console log every 2 times? the example used random numbers?
console.log(2);
console.log(4);
console.log(6);
console.log(8);
console.log(10);
console.log(12);
console.log(14);
console.log(16);
console.log(18);
console.log(20);
console.log(22);
console.log(24);
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
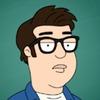
Unsubscribed User
15,444 PointsJust taken a look at the actual code challenge rather than solving the issue in my REPL.
The HTML doesn't need to be touched. You just need to replace all the console.log lines in the js file with the for loop.
In my example above I defined the variable using the let keyword, although this is best practice, the code challenge only passes if you use var, So the "correct" answer is:
for (var i = 2; i <= 24; i += 2) {
console.log( i );
}
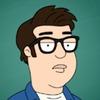
Unsubscribed User
15,444 PointsSo the best way to complete this task is to use a for loop.
The syntax for a for loop is as follows:
for (statement 1; statement 2; statement 3) {
// code block to be executed
}
In the first statement
, we want to define our variable. As this is an integer, we'll call this i. This is executed once. As this integer will increase, we would need to use a let variable.
In the second statement
, we want to declare our condition. Here we want to stop printing to the console when the integer is greater than or equal to 24.
In the third statement
we want to increase our integer by 2 every time the code block has been executed. This will run until the condition in the second statement becomes false.
for (
let i = 2; //Define variable.
i <= 24; //Declare condition
i += 2 //Increase int when code block is executed
) {
console.log( i ); //code block to be executed
}
So the answer would look like:
for (let i = 2; i <= 24; i += 2) {
console.log( i );
}

Kim Dallas
11,461 Pointsi've tried everything with this one. it still won't work. nothing in the html file references it. is that why it won't work?
Kim Dallas
11,461 PointsKim Dallas
11,461 Pointsthank you very much berian