Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial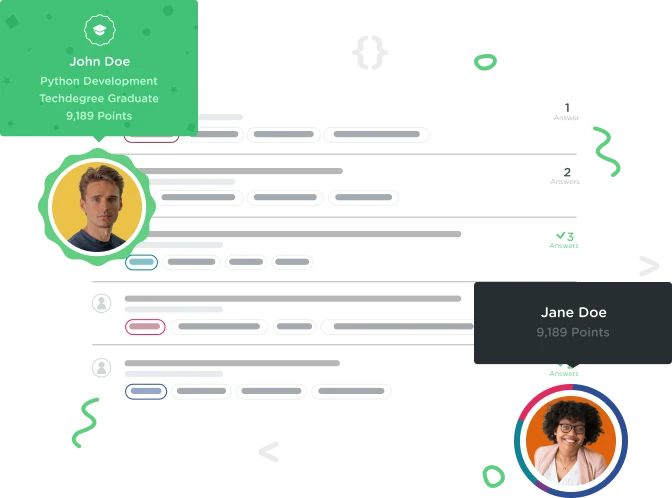

Dave Hanagan
1,360 PointsI'm confused about use of the array.filter() method at 2:40.
The filter method's anonymous function should be expecting a return of a boolean value, which determines if the array element should be included in the filtered array. But here he is explicitly returning the todo item, not a boolean. It's logical that it still works because the truthiness of the todo element still evaluates to true, but it seems unusual.
2 Answers

Thomas Nilsen
14,957 PointsIt's correct that the callback function needs to return a boolean value, but what's happening in video at 2:40 is that he returns an integer instead. This is fine because javascript can be quite helpful without you asking it to be.
Javascript knows this callback should be a boolean, so when his function return, say, the number 4, javascript will do something called type coercion and try to convert the value to a boolean value.
For example;
Boolean(4) => true
Boolean(undefined) => false
Here is a implementation of a filter function:
Array.prototype.customFilter = function (callback) {
var arr = [];
for (var i = 0; i < this.length; i++) {
//Here we call the passed in function on each element
//to determine if we should include it or not
if (callback(this[i])) {
arr.push(this[i]);
}
}
return arr;
}
var arr = [1, 2, 3, 4];
var newArr = arr.customFilter(function (n) {
if(n % 2 == 0) {
//Instead of returning n
//You could return true - that would give the same result
return n;
}
});
console.log(newArr);

Chris Dyer
18,518 PointsHey Dave! I see what you mean. This seemed unusual to me too. It's seems far more natural to use the syntax below. To each their own I guess :).
$scope.todos.filter(function(todo) { return todo.edited; })