Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial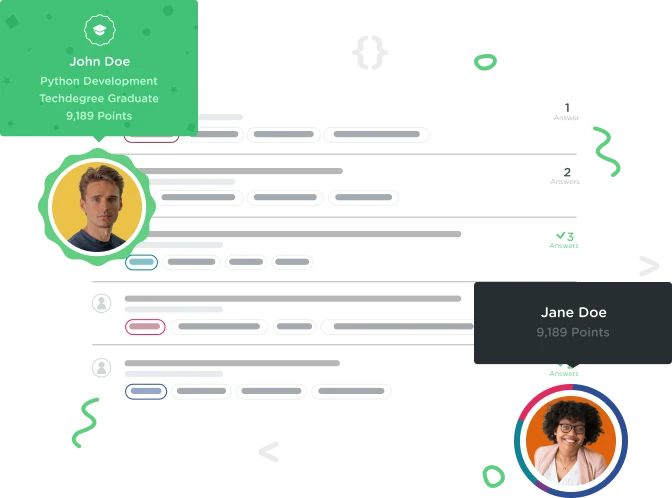

Dan Sutherland
1,463 PointsIm confused by ! operators and booleans? just can't get my head around how they work in do while loops.
I was doing the do while loop course on Javascript and in the simple game where the user guesses the number, the project uses a ! operator to break out of the do while loop when the player guesses correctly but I cannot follow it.
The variable is set outside of the loop as
var correctGuess = false;
to start with, but then within the do while loop, if the player guesses correctly then
correctGuess = true;
I understand all of this, but then the while loop argument is then set as
while ( ! correctGuess)
in order to break out if the player has guessed correctly.
Why would the while loop not just have
while ( correctGuess !==true)
I just can't understand whats going on with the Boolean and the operator to cause the loop to finish?
3 Answers

andren
28,558 PointsTo answer your question I'll first explain how the condition of a while
statement really works.
When you provide a condition to a while
statement all it cares about is whether that condition evaluates to true
or false
. If it evaluates to true
it runs, if it evaluates to false
it does not run. When you perform a comparison that operation actually results in a Boolean
value that reflects the result. So 5 > 3
becomes true
, 2 === 10
becomes false
and so on. This means that the while
statement does not really look at and care about comparisons, it only looks at the Boolean
value it ultimately receives. This holds true for other conditional statements like if
as well.
The second thing I'll explain is what the !
operator does. It's actually pretty simple, it takes whatever Boolean
follows it and returns the opposite Boolean
value. So !true
evaluates to false
, !false
evaluates to true
. That is all the operator does.
That means that when the program starts out (with correctGuess
equal to false
) the !correctGuess
condition evaluates to true
. Since the while
loop only cares about whether it is passed true
or false
this is an acceptable value for it to receive and it runs. Once correctGuess
is set equal to true
within the loop the !correctGuess
condition will now evaluate to false
(since it always becomes the opposite of the Boolean
it is used on) and once the while
statement receives false
as its condition it stops running.
The exact same events would unfold if the condition was correctGuess !== true
since that in effect also makes a true
value evaluate to false
and vice versa, but it is far more verbose than just using !correctGuess
.

Dan Sutherland
1,463 PointsAndren thank you very much for taking the time to explain that to me, it makes a lot more sense now.
Much appreciated.

benyoungblood
10,062 PointsThis is still confusing to me. I get what you are saying andren .
I'm going to type it out real quick as I process it through my own mind. Typing it out will help me. Maybe someone else as well.
The while loop is only going to run until ! correctGuess is met. Therefore, correctGuess is set to false, ! correctGuess means true, so the while statement is waiting on ! correctGuess to happen. Once it sees that correctGuess has been set to true, then ! correctGuess becomes false.
Similarly:
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt('Guess a number between 1 and 10');
guess = parseInt(guess);
guessCount +=1;
}while(guess !== randomNumber)
In this case, guess !== randomNumber is true until the user guesses the random number. Once the user guesses the random number, guess !== randomNumber becomes false, because that while statement is no longer true. So the program exits on a false statement.
Okay, I think that helped me figure it out.
George Columbu
2,076 PointsGeorge Columbu
2,076 Pointsandren Absolutely the best answer i've ever seen, i had the exact same problem. You pointed exactly what i wasnt sure about. Thank you sir!