Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial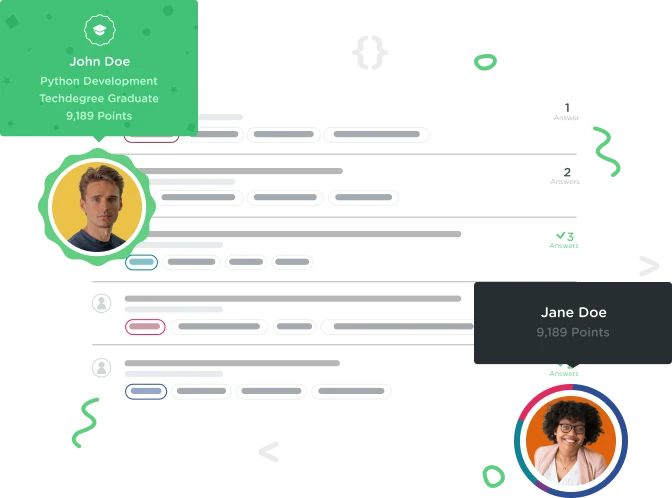
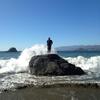
Joe Williams
4,014 PointsI'm confused by the most basic premise of JavaScript functions.
In the previous lesson, Dave taught us the most elementary concept of JavaScript functions, and I realized I'm missing something.
function getYear () {
var year = new Date().getFullYear();
return year;
}
yearToday = getYear()
I'm not 100% I understand what's happening above. Why do I have to set year = newDate().getFullYear() and then return it? That seems redundant. I've already set the var year -- why do I also have to return it?
Also, when it's all over, I don't see how yearToday = getYear() is actually calling the function, since it seems I'm only setting it equal to it.
3 Answers

Llewellyn Collins
Courses Plus Student 2,786 Points1) Why do I have to set year = newDate().getFullYear() and then return it?
All code within a function is within a code block ie the { }. Code within the block is only accessible within the block. year = newDate().getFullYear()
assigns the year value to the year variable. You need to return it so when the method is called the year value will be assigned to the yearToday variable, if you don't return it yearToday will still be undefined.
2) I don't see how yearToday = getYear() is actually calling the function.
You are not setting yearToday equal to the getYear function. by adding the parenthesis ie () after the function name, you are now calling the function. And when you call a function you execute whats within the function. And what it is doing is returning the "year" so now yearToday is equal to what is return in the getYear function which is the "year" (new Date().getFullYear()).
Hope this helps.
We use functions so we don't have to repeat code everywhere. So in this example if you wanted to create a new variable "currentYear" you can assign is as follows currentYear = getYear(); which will return the "year" instead of having to type currentYear = new Date().getFullYear();
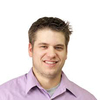
Kevin Korte
28,149 PointsWhen you set a variable to equal a function, it runs that function, and whatever the functions returns becomes the value of that variable.
Returning the variable to the function just means that the function becomes whatever that variable inside it evaluates to.
Does that help?
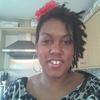
Jennifer James
6,430 PointsThank you very much for this answer, I was really stuck on the code challenge till I read this.

John Hampton
8,200 PointsI think one of the causes of confusion may be related to the Date().getFullYear()
method. The line var year = new Date().getFullYear();
is actually calling on an entirely new method, different than the getYear()
function that is the outer containing function.
The Date.getFullYear()
method will return the four-digit full year. That returned value is then assigned to var year
and is then returned by the getYear()
function.

John Hampton
8,200 PointsHere's a link to the MDN doc on the Date().getFullYear()
method: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date/getFullYear
I don't think this method was covered in the lecture, which is why it may be confusing to some.