Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial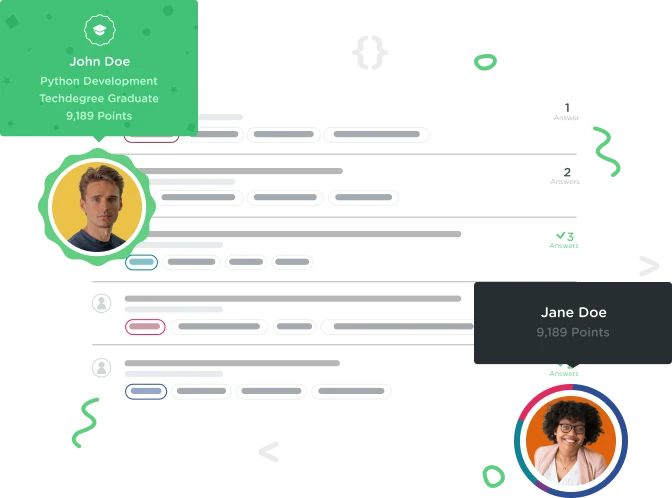

simon bao
5,522 PointsI'm confused. With the list they gave us, there is only 5 values. But the challenge expects 18 value output?
Help is appreciated!
Also if there's any improvements to code it would also be appreciated.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
dict = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(dict):
teacher_name = ""
max_count = 0
for k,v in dict.items():
if len(v) > max_count:
teacher_name = k
max_count = len(v)
return teacher_name
def num_teachers(dict):
teacher_count = 0
for k in dict.keys():
teacher_count += 1
return teacher_count
def stats(dict):
teacher_info = []
for k,v in dict.items():
teacher_info.append([k,len(v)])
return teacher_info
def courses(dict):
courses = []
for v in dict.values():
courses.append(v)
return courses
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Simon,
The challenge tester is using it's own dictionary which is different from the example given. The example is there only to show the structure of the data so that you can properly work with it.
This means that you don't need to create your own dictionary as you have done. The challenge will call all of your functions and pass in its own dictionary.
Also, since dict
is a built-in class I would use a different name for your parameter on each function. You could use my_dict
or dict1
as examples.
You almost have it for your last one. The problem is that v
in your for loop is a list and you're appending that list to your courses list. You want to extend
it instead.
Here's some output from the python shell showing the difference between these 2.
>>> my_list = [5, 3]
>>> my_list.append([4, 8])
>>> my_list
[5, 3, [4, 8]] # only has 3 items
>>> my_list = [5, 3]
>>> my_list.extend([4, 8])
>>> my_list
[5, 3, 4, 8] # has 4 items
>>>
You can either switch to extend
or you can add the lists together. courses += v
would also work.
Your 2nd and 3rd functions can be shortened down to 1 line each but there's nothing wrong with how you coded them.
As a hint for num_teachers
, the length of a dictionary is how many keys it has.
stats
can be shortened to 1 line using a list comprehension.
def stats(dict1):
return [[k,len(v)] for k,v in dict1.items()]
There's a workshop on list comprehensions if you're not familiar with them. https://teamtreehouse.com/library/python-comprehensions
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 Pointsremoved duplicate code posting