Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial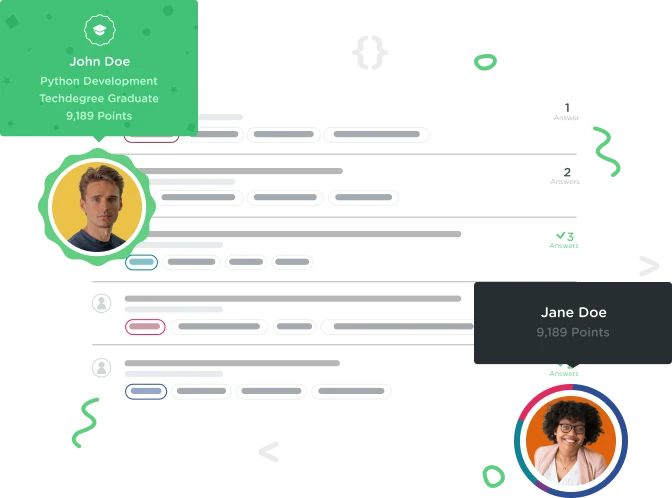

Ali Abbas
8,772 PointsI'm confused with this challenge. can anyone explain? Thanks.
I tried watching the lesson multiple times. I looked at MDN, but still can't figure it out. The challenge asks for a collection list of all items in <ul> with the id=rainbow.
var listItems = document.querySelector('[id=rainbow]');
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
1 Answer
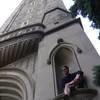
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsHey Ali, don't sweat it. Collections are quite simple. They are very similar to arrays, and they can be looped over with for
loops just like arrays can, but the main difference is that they contain DOM nodes. To get a collection of elements, you can use the children
property after you select an element from the page that has child elements. Your use of querySelector
is fine, but using getElementById
would be a bit more appropriate. Here's what you need:
var listItems = document.getElementById('rainbow').children;
Like I said, using the children
property gives you the collection of all child elements in the ul
with the ID of "rainbow".
Beware when working with collections a lot: even though they are similar to arrays, they are not arrays. You cannot use most array methods on them and you can't iterate over them using loops other than a for
loop. If you find yourself working with HTML collections a lot and you want to be able to use array methods, you can actually convert a collection to an array like this:
const foo = Array.from(collection);
Good luck and happy coding!
Ali Abbas
8,772 PointsAli Abbas
8,772 PointsYour answer was very helpful. Thank you so much for the tip as well. Now its all clear to me.
Rafael Aponte
1,328 PointsRafael Aponte
1,328 PointsExcellent answer, Michael Cook. Your answer clarified the problem for me when I was struggling.