Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial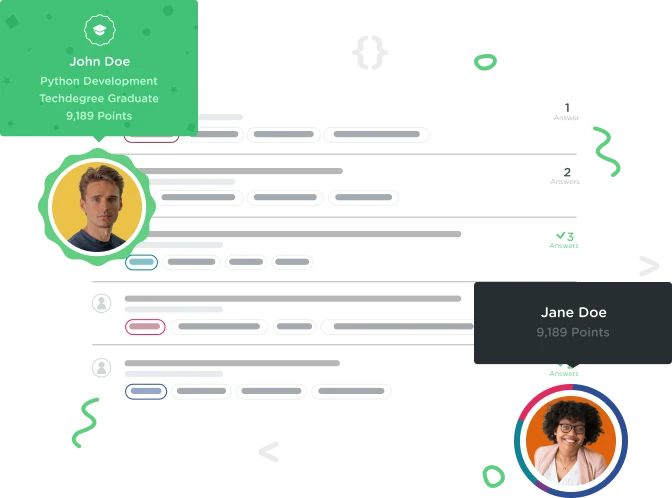

Lana Wong
3,968 PointsI'm copying the code, but still not working
I'm copying the code Pasan did in this video, and I can't find out what is wrong with my code in Xcode.
import Foundation
enum VendingSelection {
enum VendingSelection {
case soda
case dietSoda
case chips
case cookie
case sandwich
case wrap
case candyBar
case popTart
case water
case fruitJuice
case sportsDrink
case gum
}
}
protocol VendingItem {
var price: Double {get }
var quantity: Int {get set}
}
protocol VendingMachine {
var selection: [VendingSelection] { get }
var inventory: [VendingSelection: VendingItem] { get set }
var amountDeposited: Double { get set }
init(inventory: [VendingSelection: VendingSelection])
func vend(_ quantity: Int,_ selection: VendingSelection) throws
func deposite(_ amount: Double)
}
struct Item: VendingItem{
let price: Double
var quantity: Int
}
class FoodVendingMachine: VendingMachine {
let selection: [VendingSelection] = [.soda, .dietSoda, .chips, .cookies, .wrap, .sandwich, .candyBar, .poptart, .water, .fruitJuice, .sportsDrink, .gum]
var inventory: [VendingSelection: VendingItem]
var amountDeposited: Double = 10.0
required init(inventory: [VendingSelection : VendingSelection]) {
self.inventory = inventory
}
func vend(_ quantity: Int, selection: VendingSelection) throws {
}
func deposit( _ amount: Double) {
}
}
2 Answers
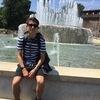
Mitch Little
11,870 PointsHi Lana,
I assume it is because you have created an enum from within another enum 'Vending Selection'.
Try:
enum VendingSelection {
case soda
case dietSoda
case chips
case cookie
case sandwich
case wrap
case candyBar
case popTart
case water
case fruitJuice
case sportsDrink
case gum
}
I hope this works,
All the best,
Mitch

Adam O' Ceallaigh
2,962 PointsHi Lana I doubt you haven't figured it out by now but in the above example the method inside the protocol Vending Machine says deposite and the function call in the class FoodVendingMachine says deposit and it won't conform to the protocol because of this because you don't have all the methods properly adhered to.
Lana Wong
3,968 PointsLana Wong
3,968 PointsOk, I fixed it and a few other problems, but I still get errors. Can you please help me solve them. I really appreciate it! :)