Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial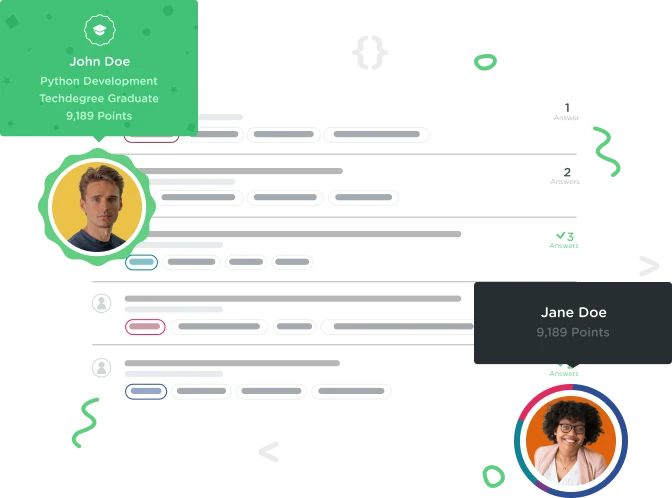

Tim Cullen
647 PointsI'm curious as what exactly the java compiler thinks is wrong with this code.
Even though it appears to do exactly what the instructions ask. As we see one overloaded member function named 'drive' is calling the other with the appropriate argument.
Bummer! Remember that you should call the existing drive method and pass in the default parameter of 1. Otherwise you would have to rewrite all that (omitted) code.
public void drive(int laps) { // Other driving code omitted for clarity purposes mBarsCount -= laps; }
public void drive() { drive( 1 ); }
6 Answers

Tim Cullen
647 PointsSo this code works perfectly fine with JDK8 on Linux. But not on the teamtreehouse site. Is this perhaps a bug in their sites runtime examination of code?
public class B { private int mBarsCount;
public B() {
mBarsCount = 8;
}
public void drive( int laps ) {
mBarsCount -= laps;
}
public void drive() {
drive( 1 );
}
}
public class A { public static void main( String[] args ) { B b = new B(); b.drive();
return;
}
}

Craig Dennis
Treehouse TeacherTry the drive(1) without spaces? It looks sane, might be my test? On my phone now or I'd check.

Tim Cullen
647 PointsOk thats really annoying. drive(1) works but drive( 1 ) doesn't work. Thats a serious parsing bug in your examination engine and/or compiler.

Craig Dennis
Treehouse TeacherYeah sorry about that. Regular Expression was needed and I messed up. Sorry for the frustration, I will get it fixed. Thanks for reporting it!

Tim Cullen
647 PointsWhile we're on the subject of parsing, how about this bit of confusion:
public void drive(int laps) { if((mBarsCount - laps) < 0) { throw IllegalArgumentException("Not enough battery remains"); } // Other driving code omitted for clarity purposes mBarsCount -= laps; }
which produces the compile time error:
./GoKart.java:21: error: cannot find symbol throw IllegalArgumentException("Not enough battery remains"); ^ symbol: method IllegalArgumentException(String) location: class GoKart 1 error
Did I mistype something and just can't see it?

Craig Dennis
Treehouse TeacherYou are missing the new
keyword before the exception.

Tim Cullen
647 PointsAh ha, so unlike C++ you have to dynamically allocate an exception object at the point of throwing that exception. These are exactly the little differences I'm looking for. thanks.
Tim Cullen
647 PointsTim Cullen
647 PointsIt appears that the copy and paste didn't work out so well. In reality the statement "mBarsCount -= laps" is not on the same line as the comment and is therefore not actually commented out as it appears here.