Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial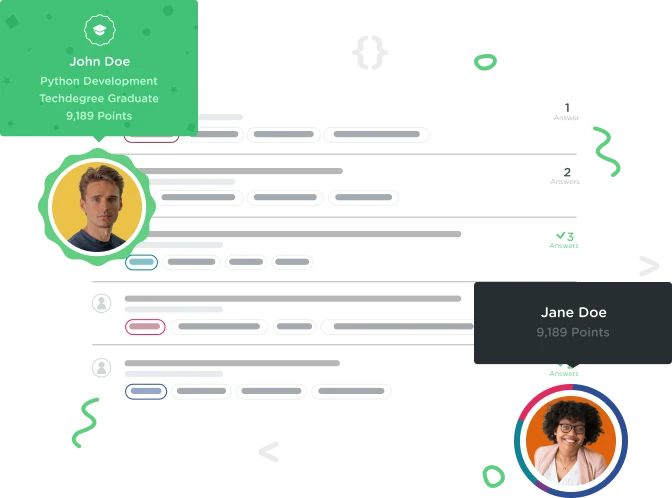
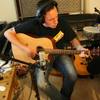
Benjamin Gooch
20,367 PointsI'm doing this differently... Is there any issues with the way I'm doing it that I'm not foreseeing?
So I've taken to putting most of the System.out stuff into the methods themselves inside the Object (PezDispenser) instead of in the Example.java code.
I think it ties the actions and results to the object and their methods, instead of the Example.java file. Here's my code:
Example.java:
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new PEZ Dispenser");
//System.out.printf("FUN FACT: There are %d PEZ allowed in every dispenser. %n",
// PezDispenser.MAX_PEZ);
//Create new PezDispenser object
PezDispenser dispenser = new PezDispenser();
//Print to console the name of the dispenser character (characterName)
System.out.printf("The dispenser is %s. %n",
dispenser.getCharacterName()
);
//Tells the user the dispenser is empty
if (dispenser.isEmpty() == true) {
System.out.println("The dispenser is empty.");
} else {
System.out.println("The dispenser still has some in there!");
}
//Call fill() method to set dispenser to full, or MAX_PEZ
dispenser.fill();
}
}
and PezDispenser.java
class PezDispenser {
//Define max PEZ allowed in dispenser
public static final int MAX_PEZ = 12;
//Declaring member variables
final private String characterName;
private int pezCount;
//PezDispenser constructor, uses name passed in the method when called to set characterName, sets PEZ count to zero, as they come empty
public PezDispenser(String name) {
this.characterName = name;
pezCount = 0;
}
//Method returns name of character (characterName) when called
public String getCharacterName() {
return characterName;
}
//Method sets the pezCount member variable to 12, which is the MAX_PEZ that a dispenser can hold at a time
public void fill() {
System.out.printf("Filling your %s PEZ dispenser with delicious PEZ! %n",
getCharacterName()
);
pezCount = MAX_PEZ;
}
//Boolean return on whether or not the dispenser is empty
public boolean isEmpty() {
return pezCount == 0;
}
//Method returns the quantity of PEZ in the dispenser
public String getPezCount(){
return "Your " + characterName + " dispenser currently has " + pezCount + " PEZ remaining.";
}
//Method "eats" one PEZ brick
public void eat(){
if (pezCount > 0) {
pezCount -= 1;
System.out.println("Yummy! You just ate one PEZ brick.");
} else {
System.out.println("You cannot eat what isn't there! Try filling it first.");
}
}
}
Is there some reason you'd not want to do this?
Thanks!!
1 Answer

shashank hk
1,931 PointsHi, I think below code is redundant if (dispenser.isEmpty() == true) { System.out.println("The dispenser is empty."); }
because "if" will be evaluated only if its Boolean condition results to be True intrernally .So you don't need to explicitly check if its true or false
shrusha shende
1,156 Pointsshrusha shende
1,156 PointsI dont think so I did the same thing