Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial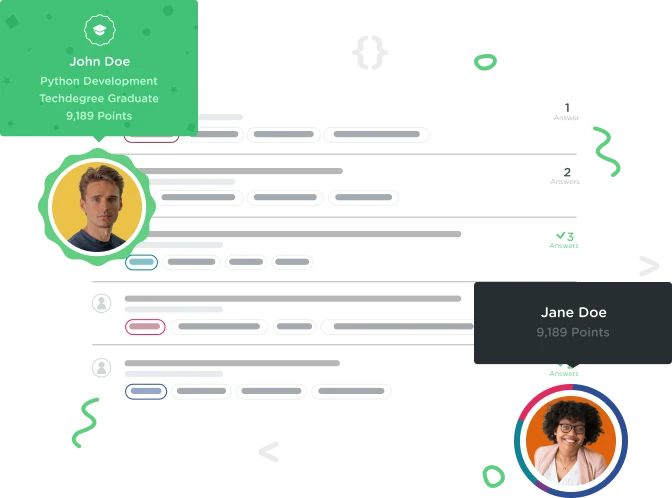
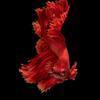
Michael Williams
Courses Plus Student 8,059 PointsI'm failing to see the usefulness of the parentNode property. Can someone explain it better?
I know there are so many different ways to solve a single problem in JavaScript. However, I just don't see the value in the .parentNode
property yet. For example, here's a chunk of code we wrote in the video:
listUl.addEventListener("click", (event) => {
if (event.target.tagName == "BUTTON") {
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
});
Why not just loop through and delete with an if
statement instead? That seemed easier to write and conceptually easier to understand.
2 Answers
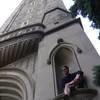
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsThe JavaScript browser environment gives you a lot of different tools to use to control your HTML. It's hard to think of a specific example off the top of my head, but there probably are going to be situations in which it is actually the most efficient solution to traverse either to or from the parent node of an element. In other cases, like in the one you point out, something else might make more sense. Even if it doesn't seem particularly useful to you right now, no doubt you will encounter use cases where it is in your career if you pursue front-end application development. Therefore, I suggest using whatever DOM traversal methods are comfortable to you and seem to make sense, but at the same time be aware of other built-in methods, because there may come a point where they are going to work for you. :)
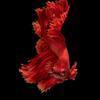
Michael Williams
Courses Plus Student 8,059 PointsThank you, Michael Cook. Slow reply on my end because of crazy work life. Anywho, thanks again for these thoughts. As I dove back in this afternoon things started clicking, and it's making sense how this is one way to provide a "map" of sorts for how to get to specific items in the DOM.
Random question, for you. How long of studying did it take you to get to where you're pretty proficient at JavaScript?
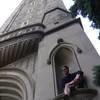
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMy pleasure. As to your question, since about mid February of this year. I began slowly by reading a beginner book and doing some tutorials online, and I took a lot of notes and that slowed me down. I gradually realized that taking notes was pointless because I rarely went back to look at them. If instead of taking notes I had just gotten used to reading the MDN web docs right away (though they are difficult) and playing around with code more than studying it, I would have made faster progress and I think that goes for just about anyone. A programming language is a tool. Carpenters don't get good at using their tools by studying them in books, they do it by using those tools and building things. Don't be discouraged if a lot of what you learn feels unfamiliar and confusing a lot of the time. Just play around with it a lot and you will notice the pace at which you learn will start to accelerate. :)
Michael Williams
Courses Plus Student 8,059 PointsMichael Williams
Courses Plus Student 8,059 PointsThank you. I guess after all that, I still don't understand them completely. Like I understand what's going on, but I feel like I need a deeper explanation from the videos than what's provided (which is currently very surface level). To put it more succinctly, it feels like the explanations are a little geared toward people who may understand the concepts a little and not someone like me, who's completely new to the concepts.
Would you happen to have any resources that explain it better/on a deeper level? Thanks!
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsHey Michael, today I can think of a common example in which you would want to use the parentNode method. Consider a navigation bar that is made of an unordered list. It might look like this:
<ul> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Portfolio</a></li> <li><a href="#">Github</a></li> </ul>
Now let's say that you want to add some kind of effect to each list item each time the user clicks the link inside the list item. Perhaps you want to change the colour of the link, or some other effect. You could do this easily with CSS, but for the sake of the example, suppose you wanted to do it with JavaScript. Your JS would then look something like this:
document.querySelector('ul').on('click', function(event) { if (event.target.tagName == 'A') { event.target.parentNode.style.backgroundColor = 'purple'; }; });
This might seem like a very trivial example, and it is, but it does serve to demonstrate how in a certain situation the parentNode property could be quite useful.
In terms of resources to help you understand better, the Mozilla Developer Network web docs are really the best source of information. They are tough to read and I still have some difficulty with them as well, but the more familiar you get with them the easier they become to understand and the more you will find yourself naturally going to them to read about something. The page for parentNode is https://developer.mozilla.org/en-US/docs/Web/API/Node/parentNode. Also, I personally found it very helpful to read a beginner book on JavaScript before I ever signed up for Treehouse. I read the book JavaScript for Kids: A Playful Introduction to Programming by Nick Morgan, published by No Starch Press. This book is great for gifted children or adults, but it doesn't cover much DOM scripting. But there are lots of books out there on the market, and the great thing about books is that they are entirely self-paced. You can re-read a line as many times as you want without having to re-wind and find the exact spot where you started to misunderstand.
Just keep working at this stuff and it is going to become more familiar and intuitive, guaranteed.