Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial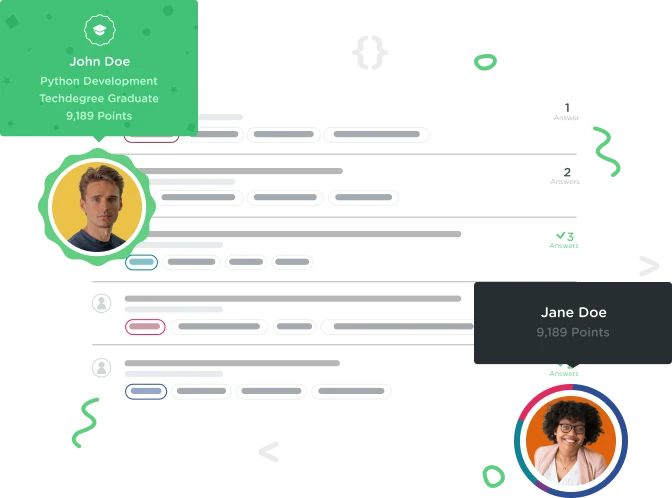
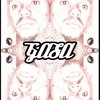
MUZ140750 Bhekimpi Sithole
2,640 PointsIm failing to understand where l am making an error, please help
when l run the code it says it could not get all the twitter handles
import re
string = '''Love, Kenneth, kenneth+challenge@teamtreehouse.com, 555-555-5555, @kennethlove
Chalkley, Andrew, andrew@teamtreehouse.co.uk, 555-555-5556, @chalkers
McFarland, Dave, dave.mcfarland@teamtreehouse.com, 555-555-5557, @davemcfarland
Kesten, Joy, joy@teamtreehouse.com, 555-555-5558, @joykesten'''
contacts = re.search(r''' #<-- Wrap in multiline quote. Remove leading caret (^)
(?P<email>[-\w\d.+]+@[-\w\d.]+)
,\s
(?P<phone>\d{3}-\d{3}-\d{4})''' #<-- Add closing paren. Remove extra backslashes. Remove trailing $
, string, re.X | re.M) #<-- Add verbose (for multiline quote), and multiline (for multiple lines in string) flags
twitters = re.search(r'''
(?P<twitters>@[\w\d]+)?$
''', string, re.X | re.M)
2 Answers

Unsubscribed User
3,273 PointsLets just clean up your code a bit first:
import re
string = '''Love, Kenneth, kenneth+challenge@teamtreehouse.com, 555-555-5555, @kennethlove
Chalkley, Andrew, andrew@teamtreehouse.co.uk, 555-555-5556, @chalkers
McFarland, Dave, dave.mcfarland@teamtreehouse.com, 555-555-5557, @davemcfarland
Kesten, Joy, joy@teamtreehouse.com, 555-555-5558, @joykesten'''
contacts = re.search(r'''
(?P<email>[+-\w\d.]+@[-\w\d.]+) ,\s
(?P<phone>[\d]{3}-[\d]{3}-[\d]{4})
''', string, re.X | re.M)
twitters = re.search(r'(?P<twitters>@[\w\d]+)$', string, re.M)
So here are the changes I made:
1) Removed comments to increase readability
2) Moved ,\s to the same line as email outside of the capture group
3) Added brackets around the \d calls in the phone capture group for readability
4) Moved final ''' of the contacts variable to the same line as, string
5) Reformatted the twitters variable to be on a single line since there is no need to be verbose with only 1 capture group.
6) Removed re.X flag from twitters since it is no longer verbose
I tested this code and it is a functioning answer.

Unsubscribed User
3,273 PointsYes, that is exactly it. The $ character tells the regex to look at the end of the line when paired with re.M. Otherwise it just looks as the end of the word.
Andrew Gordon
10,178 PointsAndrew Gordon
10,178 PointsSo I have a question for your answer.
I utilized the same code for the twitter section:
twitters = re.search(r'(?P<twitters>@[\w\d]+)', string, re.M
But I did not add the '$' at the end like what you have. It seems that was all I was missing for my answer to be correct, but I don't understand why. Is the '$' the escape character that tells the regex to check the end of a line for the twitter? I'd love some input on this.
Thanks! Andrew