Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial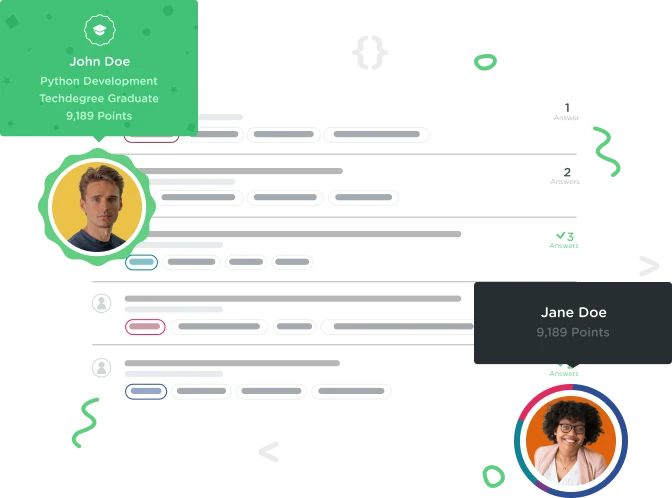
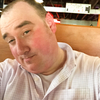
Victor Curtis Jr
5,268 PointsI'm following the code in the video but getting errors that I'm not sure how to fix!
I'm carefully following the instructor's code in the video, but I'm getting errors and I'm not sure what I'm doing wrong.
Example class:
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new PEZ dispenser");
PezDispenser dispenser = new PezDispenser("Donatello");
System.out.printf("The dispenser is %s %n",
dispenser.getCharacterName());
String before = dispenser.swapHead("Darth Vader");
System.out.printf("It was %s but Chris switched it to %s %n",
before,
dispenser.getCharacterName());
}
}
PezDispenser class:
class PezDispenser {
private String characterName = "Yoda";
public PezDispenser(String characterName) {
this.characterName = characterName;
}
public String getCharacterName() {
return characterName;
public String swapHead(String characterName) {
String originalCharacterName = this.characterName;
this.characterName = characterName;
return originalCharacterName;
}
}
}
The compiler errors:
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
Picked up _JAVA_OPTIONS: -Xmx128m
./PezDispenser.java:12: error: illegal start of expression
public String swapHead(String characterName) {
^
./PezDispenser.java:12: error: ';' expected
public String swapHead(String characterName) {
^
./PezDispenser.java:12: error: ';' expected
public String swapHead(String characterName) {
^
Example.java:10: error: cannot find symbol
String before = dispenser.swapHead("Darth Vader");
^
symbol: method swapHead(String)
location: variable dispenser of type PezDispenser
4 errors
3 Answers
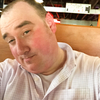
Victor Curtis Jr
5,268 PointsThank you so much!! It actually was an extra }. Duh. Thank you so much for your help! :)
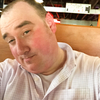
Victor Curtis Jr
5,268 PointsSo I found the curly brace that I forgot. So I added that at the end of the getCharacterName method. This solved most of the errors, but I'm still getting one error.
treehouse:~/workspace$ javac Example.java
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
Picked up _JAVA_OPTIONS: -Xmx128m
./PezDispenser.java:19: error: class, interface, or enum expected
}
^
1 error

Craig Dennis
Treehouse TeacherMaybe an extra }
?

Craig Dennis
Treehouse TeacherSomething is missing at the end of your getCharacterName
method definition...
Let me know if that hint doesn't do it.
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherOnly reason I can see it is because I've made the same mistakes! Great job sticking with it!