Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial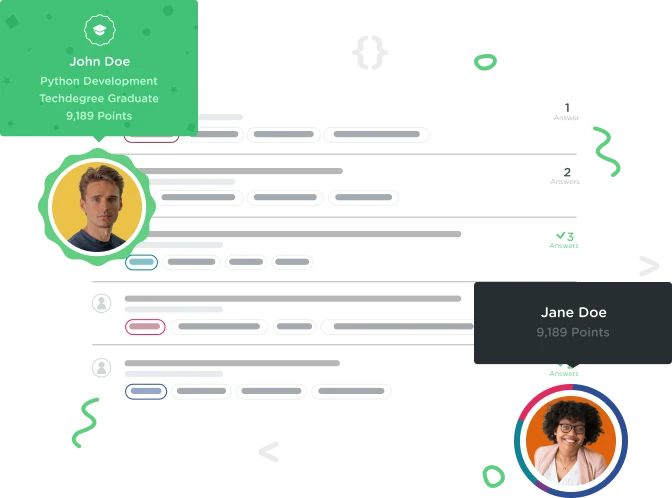

Diego Marrs
8,243 PointsI'm forgetting the proper import statements?
So i'm currently taking the challenge for 'sets'...I imported all that was necessary but it still tells me that I forgot to add the proper import statements.
package com.example;
import java.util.List;
import java.util.Set;
import java.util.HashSet;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> allPosts = new TreeSet<String>();
for (String word : getPosts()) {
if (word.equals(getAuthor())) {
allPosts.getAuthor(word);
}
}
return allPosts;
}
}

Simon Coates
28,694 PointsJeremy Hill notes a type mismatch. getPost returns a lits of BlogPost, which can't be assigned to the String word. Beyond this, you seem confused.
if (word.equals(getAuthor())) {
with the above, get author is being called, but not on anything. getAuthor is a BlogPost method, hence has to be called on an instance of a blogpost.
allPosts.getAuthor(word);
with the above, allPosts is a set of strings, hence probably doesn't have a getAuthor method.
(The message about import statements seems to be happening because the code finds something it can't understand, and assumes that you forget to import something. I think.)
4 Answers
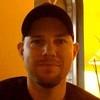
Jeremy Hill
29,567 PointsI just jumped into the challenge and passed with this code, but don't forget to add imports for Set and TreeSet:
//import these at the top under your package declaration:
//import java.util.Set;
//import java.util.TreeSet;
public Set<String> getAllAuthors(){
Set<String> authors = new TreeSet<String>();
for(BlogPost post : mPosts){
authors.add(post.getAuthor());
}
return authors;
}

Diego Marrs
8,243 PointsAh, it was so obvious! :(
We weren't suppose to make a list of the posts, but a list of the authors! That explains...everything! Thank you so much!
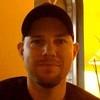
Jeremy Hill
29,567 PointsYea your for loop needs to look like this:
for(BlogPost post : mPosts)
or at least something along those lines. and when you are using the methods from class BlogPost it should look like this:
post.getAuthor()
That is if it is inside your for loop.

Diego Marrs
8,243 PointsSo, I read your comment and edited my code...but now i'm super confused where to go from here and if I still need the 'allPosts' list.
public Set<String> getAllAuthors() {
Set<String> allPosts = new TreeSet<String>();
for (BlogPost post : mPosts) {
if (post.equals(post.getAuthor())) {
}
}
return allPosts;
}
This also works:
for (String word : allPosts) {
}

Simon Coates
28,694 PointsIf you read through the other answers and haven't had any luch, try
public Set<String> getAllAuthors() {
Set<String> allPosts = new TreeSet<String>();
for (BlogPost bp : this.getPosts()) {
allPosts.add( bp.getAuthor());
}
return allPosts;
}
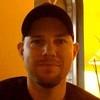
Jeremy Hill
29,567 PointsYea lol sometimes I miss those little details as well. Happy coding :)
Philip Gales
15,193 PointsPhilip Gales
15,193 PointsHave you looked at the compiler messages? It looks like it does not like your for each loop.