Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial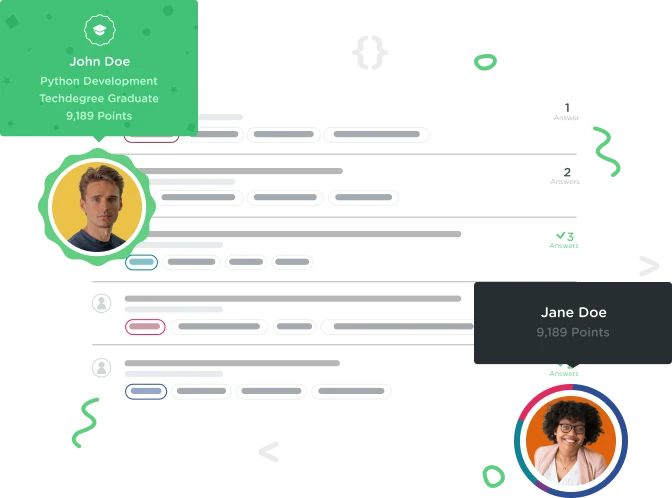

Yan Kozlovskiy
30,427 PointsI'm getting a 301 HTTP response code instead of a 200. What should I do to fix the problem?
The 301 response states that the page has been moved permanently. I confirmed this by going to the URL with my browser. The browser redirected to the HTTPS version of the page. However every time I try to load the HTTPS version of that page, by running node, the console throws an error.
With HTTP in the code:
//console.log("Hello, world!");
//var name = "Andrew";
//var details = { favouriteLanguage: "JavaScript", age: 31, children: 2.4 }
//var errorMessage = "Something bad has occurred";
//
//console.log(name);
//console.log(details);
var http = require('http');
var username = 'chalkers';
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in JavaScript";
console.log(message);
}
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response) {
console.log(response.statusCode);
});
request.on('error', function(error){
console.error(error.message);
});
treehouse:~/workspace$ node app.js
301
With HTTPS in the code:
//console.log("Hello, world!");
//var name = "Andrew";
//var details = { favouriteLanguage: "JavaScript", age: 31, children: 2.4 }
//var errorMessage = "Something bad has occurred";
//
//console.log(name);
//console.log(details);
var http = require('http');
var username = 'chalkers';
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in JavaScript";
console.log(message);
}
var request = http.get("https://teamtreehouse.com/" + username + ".json", function(response) {
console.log(response.statusCode);
});
request.on('error', function(error){
console.error(error.message);
});
treehouse:~/workspace$ node app.js
_http_client.js:75
throw new Error('Protocol "' + protocol + '" not supported. ' +
^
Error: Protocol "https:" not supported. Expected "http:".
at new ClientRequest (_http_client.js:75:11)
at Object.exports.request (http.js:49:10)
at Object.exports.get (http.js:53:21)
at Object.<anonymous> (/home/treehouse/workspace/app.js:16:20)
at Module._compile (module.js:460:26)
at Object.Module._extensions..js (module.js:478:10)
at Module.load (module.js:355:32)
at Function.Module._load (module.js:310:12)
at Function.Module.runMain (module.js:501:10)
at startup (node.js:129:16)
5 Answers

Casey Ydenberg
15,622 Pointsrequire('https')
instead of require('http')
should do it I think

Yan Kozlovskiy
30,427 PointsIs there a difference between the HTTP and HTTPS?

Casey Ydenberg
15,622 PointsAccording to the documentation they are separate modules that expose many of the same methods. So yes, using http.get
and then providing an https
URL will throw an error.

Chan Yi
1,576 PointsTry using https on both require() and the url for the get method.
var https = require('https');
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) { console.log(response.statusCode); });
This has worked for me.

Carlos Sanchez
8,900 PointsThe video class is no longer relevant if the http has to be switched by the https, this course needs updating.
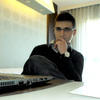
Daniel Nitu
13,272 PointsYou need to change to 'https' everywhere in the code and it should work. I also changed the variable 'http' in order to avoid confusion. I now receive the 200 statusCode.

Carl Stålhem
24,726 PointsSwitching to https no longer works. It gives a 404 Not Found status. Using http still gives a 301 status. It does not have a "location:" field with the new URL as it should so I think this has to be looked at.
Alex Deas
1,714 PointsAlex Deas
1,714 PointsCan you post relevant code please?